poolelement
This module is part of the Python Pool library. It defines the base classes for
Classes
PoolBaseElement
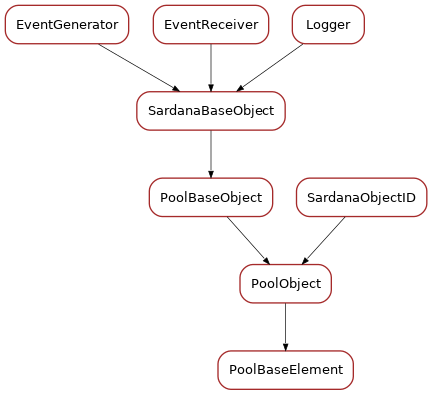
- class PoolBaseElement(**kwargs)[source]
Bases:
PoolObject
A Pool object that besides the name, reference to the pool, ID, full_name and user_full_name has:
_simulation_mode : boolean telling if in simulation mode
_state : element state
_status : element status
- get_state(cache=True, propagate=1)[source]
Returns the state for this object. If cache is True (default) it returns the current state stored in cache (it will force an update if cache is empty). If propagate > 0 and if the state changed since last read, it will propagate the state event to all listeners.
- Parameters:
- Return type:
State
- Returns:
the current object state
- inspect_state()[source]
Looks at the current cached value of state
- Return type:
State
- Returns:
the current object state
- property state: Enumeration('State', ['On', 'Off', 'Close', 'Open', 'Insert', 'Extract', 'Moving', 'Standby', 'Fault', 'Init', 'Running', 'Alarm', 'Disable', 'Unknown', 'Invalid'])
element state
- inspect_status()[source]
Looks at the current cached value of status
- Return type:
- Returns:
the current object status
- get_status(cache=True, propagate=1)[source]
Returns the status for this object. If cache is True (default) it returns the current status stored in cache (it will force an update if cache is empty). If propagate > 0 and if the status changed since last read, it will propagate the status event to all listeners.
- Parameters:
- Return type:
- Returns:
the current object status
- calculate_state_info(status_info=None)[source]
Transforms the given state information. This specific base implementation transforms the given state,status tuple into a state, new_status tuple where new_status is “self.name is state plus the given status. It is assumed that the given status comes directly from the controller status information.
PoolElement
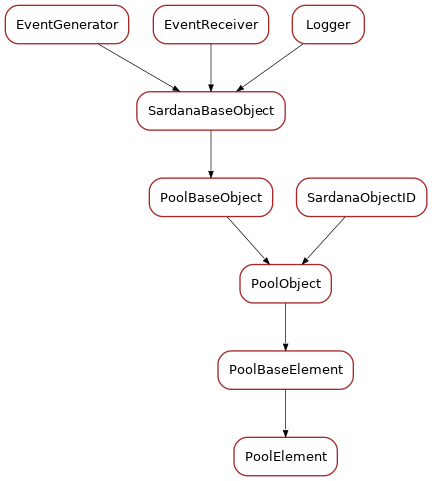
- class PoolElement(**kwargs)[source]
Bases:
PoolBaseElement
A Pool element is an Pool object which is controlled by a controller. Therefore it contains a _ctrl_id and a _axis (the id of the element in the controller).
- init_attribute_values(attr_values=None)[source]
Initialize attributes with (default) values.
Set values to attributes as passed in
attr_values
. In lack of attribute value apply default value. In lack of default value do nothing.
- get_dependent_elements()[source]
Get elements which depend on this element.
Get elements e.g. pseudo elements or groups, which depend on this element.
- Return type:
- Returns:
dependent elements
- property axis
element axis
- property controller
element controller
- property controller_id
element controller id
- property instrument
element instrument
- property deleted
element is deleted from pool (experimental API)