poolmeasurementgroup
This module is part of the Python Pool library. It defines the base classes for
Functions
Classes
- build_measurement_configuration(user_elements)[source]
Create a minimal measurement configuration data structure from the user_elements list.
Minimal configuration data structure:
dict <str, dict> with keys: - 'controllers' : where value is a dict<str, dict> where: - key: controller's full name - value: dict<str, dict> with keys: - 'channels' where value is a dict<str, obj> where: - key: channel's full name - value: dict<str, obj> with keys: - 'index' : where value is the channel's index <int>
Note
The build_measurement_configuration function has been included in Sardana on a provisional basis. Backwards incompatible changes (up to and including removal of the function) may occur if deemed necessary by the core developers.
PoolMeasurementGroup
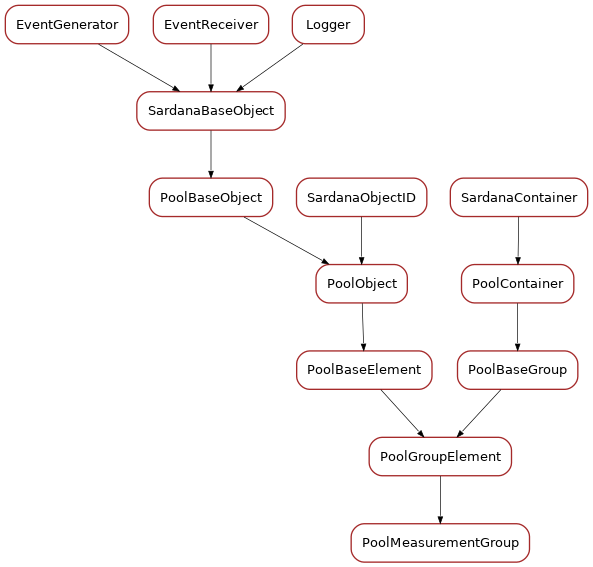
- class PoolMeasurementGroup(**kwargs)[source]
Bases:
PoolGroupElement
- init_attribute_values(attr_values=None)[source]
Initialize attributes with values.
Set values to attributes as passed in
attr_values
. In lack of attribute value do nothing.
- add_user_element(element, index=None)[source]
Override the base behavior, so the TriggerGate elements are silently skipped if used multiple times in the group
- property configuration
- property integration_time
the current integration time
- property monitor_count
the current monitor count
- property acquisition_mode
the current acquisition mode
- property synch_description
the current synchronization description
- property moveable
moveable source used in synchronization description
- property latency_time
latency time between two consecutive acquisitions
- property software_synchronizer_initial_domain
software synchronizer initial domain (SynchDomain.Time or SynchDomain.Position)
- property nb_starts
current number of starts
- prepare()[source]
Prepare for measurement.
Delegate measurement preparation to the acquisition action.
- start_acquisition(value=None)[source]
Start measurement.
Delegate start measurement to the acquisition action. Provide backwards compatibility for starts without previous prepare.
- property acquisition
acquisition object
ConfigurationItem
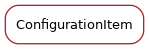
- class ConfigurationItem(element, attrs=None)[source]
Bases:
object
Container of configuration attributes related to a given element.
Wrap an element to pretend its API. Manage the element’s configuration. Hold an information whether the element is enabled. By default it is enabled.
Note
The ConfigurationItem class has been included in Sardana on a provisional basis. Backwards incompatible changes (up to and including removal of the class) may occur if deemed necessary by the core developers.
- property element
Returns the element associated with this item
ControllerConfiguration
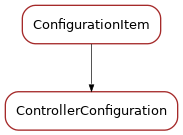
- class ControllerConfiguration(element, attrs=None)[source]
Bases:
ConfigurationItem
Container of configuration attributes related to a given controller.
Inherit behavior from
ConfigurationItem
and additionally hold information about its enabled/disabled channels. By default it is disabled.Note
The ControllerConfiguration class has been included in Sardana on a provisional basis. Backwards incompatible changes (up to and including removal of the class) may occur if deemed necessary by the core developers.
TimerableControllerConfiguration
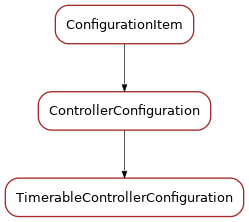
- class TimerableControllerConfiguration(element, attrs=None)[source]
Bases:
ControllerConfiguration
Container of configuration attributes related to a given timerable controller.
Inherit behavior from
ControllerConfiguration
and additionally validate timer and monitor configuration.Note
The TimerableControllerConfiguration class has been included in Sardana on a provisional basis. Backwards incompatible changes (up to and including removal of the class) may occur if deemed necessary by the core developers.
ExternalControllerConfiguration
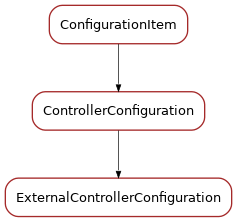
- class ExternalControllerConfiguration(element, attrs=None)[source]
Bases:
ControllerConfiguration
Container of configuration attributes related to a given external controller.
Inherit behavior from
ControllerConfiguration
.Note
The ExternalControllerConfiguration class has been included in Sardana on a provisional basis. Backwards incompatible changes (up to and including removal of the class) may occur if deemed necessary by the core developers.
ChannelConfiguration
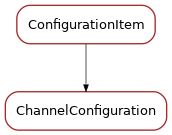
- class ChannelConfiguration(element, attrs=None)[source]
Bases:
ConfigurationItem
Container of configuration attributes related to a given experimental channel.
Inherit behavior from
ConfigurationItem
.Note
The ChannelConfiguration class has been included in Sardana on a provisional basis. Backwards incompatible changes (up to and including removal of the class) may occur if deemed necessary by the core developers.
SynchronizerConfiguration
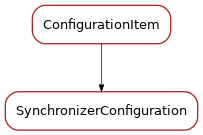
- class SynchronizerConfiguration(element, attrs=None)[source]
Bases:
ConfigurationItem
Container of configuration attributes related to a given synchronizer element.
Inherit behavior from
ConfigurationItem
. By default it is disabled.Note
The ChannelConfiguration class has been included in Sardana on a provisional basis. Backwards incompatible changes (up to and including removal of the class) may occur if deemed necessary by the core developers.
MeasurementConfiguration
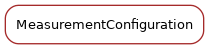
- class MeasurementConfiguration(parent=None)[source]
Bases:
object
Configuration of a measurement.
Accepts import and export from/to a serializable data structure (based on dictionaries/lists and strings). Provides getter methods that facilitate extracting of information e.g. controllers of different types, master timers/monitors, etc.
Note
The build_measurement_configuration function has been included in Sardana on a provisional basis. Backwards incompatible changes (up to and including removal of the function) may occur if deemed necessary by the core developers.
- DFT_DESC = 'General purpose measurement configuration'
- get_acq_synch_by_channel(channel)[source]
Return acquisition synchronization configured for this element.
- Parameters:
channel – channel to look for its acquisition synchronization or
ChannelConfiguration
- Returns:
acquisition synchronization
- get_acq_synch_by_controller(controller)[source]
Return acquisition synchronization configured for this controller.
- Parameters:
controller (
Union
[PoolController
,ControllerConfiguration
]) – controller to look for its acquisition synchronization orControllerConfiguration
- Return type:
- Returns:
acquisition synchronization
- get_timerable_ctrls(acq_synch=None, enabled=None)[source]
Return timerable controllers.
Allow to filter controllers based on acquisition synchronization or whether these are enabled/disabled.
- Parameters:
- Return type:
- Returns:
timerable controllers that fulfils the filtering criteria
- get_timerable_channels(acq_synch=None, enabled=None)[source]
Return timerable channels.
Allow to filter channels based on acquisition synchronization or whether these are enabled/disabled.
- Parameters:
- Return type:
- Returns:
timerable channels that fulfils the filtering criteria
- get_zerod_ctrls(enabled=None)[source]
Return 0D controllers.
Allow to filter controllers whether these are enabled/disabled.
- get_synch_ctrls(enabled=None)[source]
Return synchronizer (currently only trigger/gate) controllers.
Allow to filter controllers whether these are enabled/disabled.
- get_synchs(enabled=None)[source]
Return synchronizers (currently only trigger/gate).
Allow to filter synchronizers whether these are enabled/disabled.
- get_master_timer_software()[source]
Return master timer in software acquisition.
- Return type:
- Returns:
master timer in software acquisition
- get_master_monitor_software()[source]
Return master monitor in software acquisition.
- Return type:
- Returns:
master monitor in software acquisition
- get_master_timer_software_start()[source]
Return master timer in software start acquisition.
- Return type:
- Returns:
master timer in software start acquisition
- get_master_monitor_software_start()[source]
Return master monitor in software start acquisition.
- Return type:
- Returns:
master monitor in software start acquisition
- set_configuration_from_user(cfg)[source]
Set measurement configuration from serializable data structure.
Setting of the configuration includes the validation process. Setting of invalid configuration raises an exception and leaves the object as it was before the setting process. Thanks to that it is not necessary that the client application does the validation.
The configuration parameters for given channels/controllers may differ depending on their types e.g. 0D channel does not support timer parameter while C/T does.
Todo
Raise exceptions when setting _Synchronization_ parameter for external channels, 0D and PSeudoCounters.