sardanamodulemanager
This module is part of the Python Sardana library. It defines the base classes for module manager
Classes
ModuleManager
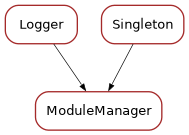
- class ModuleManager(*p, **k)[source]
This class handles python module loading/reloading and unloading.
- loadModule(module_name, path=None)[source]
Loads the given module name. If the module has been already loaded into this python interpreter, nothing is done.
- Parameters:
- Return type:
- Returns:
python module
- Raises:
ImportError
- Critical = 50
Critical message level (constant)
- Debug = 10
Debug message level (constant)
- DftLogFormat = <logging.Formatter object>
Default log format (constant)
- DftLogLevel = 20
Default log level (constant)
- DftLogMessageFormat = '%(threadName)-14s %(levelname)-8s %(asctime)s %(name)s: %(message)s'
Default log message format (constant)
- Error = 40
Error message level (constant)
- Fatal = 50
Fatal message level (constant)
- Info = 20
Info message level (constant)
- Trace = 5
Trace message level (constant)
- Warning = 30
Warning message level (constant)
- addChild(child)
Adds a new logging child
- Parameters:
child (logging.Logger) – the new child
- classmethod addLevelName(level_no, level_name)
Registers a new log level
- addLogHandler(handler)
Registers a new handler in this object’s logger
- Parameters:
handler (logging.Handler) – the new handler to be added
- classmethod addRootLogHandler(h)
Adds a new handler to the root logger
- Parameters:
h (logging.Handler) – the new log handler
- call__init__(klass, *args, **kw)
Method to be called from subclasses to call superclass corresponding __init__ method. This method ensures that classes from diamond like class hierarquies don’t call their super classes __init__ more than once.
- call__init__wo_kw(klass, *args)
Same as call__init__ but without keyword arguments because PyQt4 does not support them.
- copyLogHandlers(other)
Copies the log handlers of other object to this object
- Parameters:
other (object) – object which contains ‘log_handlers’
- critical(msg, *args, **kw)
Record a critical message in this object’s logger. Accepted args and kwargs* are the same as
logging.Logger.critical()
.- Parameters:
msg (str) – the message to be recorded
args – list of arguments
kw – list of keyword arguments
- debug(msg, *args, **kw)
Record a debug message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.debug()
.- Parameters:
msg (str) – the message to be recorded
args – list of arguments
kw – list of keyword arguments
- deprecated(msg=None, dep=None, alt=None, rel=None, dbg_msg=None, _callerinfo=None, **kw)
Record a deprecated warning message in this object’s logger. If message is not passed, a estandard deprecation message is constructued using dep, alt, rel arguments. Also, an extra debug message can be recorded, followed by traceback info.
- Parameters:
msg (str) – the message to be recorded (if None passed, it will be constructed using dep (and, optionally, alt and rel)
dep (str) – name of deprecated feature (in case msg is None)
alt (str) – name of alternative feature (in case msg is None)
rel (str) – name of release from which the feature was deprecated (in case msg is None)
dbg_msg (str) – msg for debug (or None to log only the warning)
_callerinfo – for internal use only. Do not use this argument.
kw – any additional keyword arguments, are passed to
logging.Logger.warning()
- classmethod disableLogOutput()
Disables the
logging.StreamHandler
which dumps log records, by default, to the stderr.
- classmethod enableLogOutput()
Enables the
logging.StreamHandler
which dumps log records, by default, to the stderr.
- error(msg, *args, **kw)
Record an error message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.error()
.- Parameters:
msg (str) – the message to be recorded
args – list of arguments
kw – list of keyword arguments
- exception(msg, *args)
Log a message with severity ‘ERROR’ on the root logger, with exception information.. Accepted args are the same as
logging.Logger.exception()
.- Parameters:
msg (str) – the message to be recorded
args – list of arguments
- fatal(msg, *args, **kw)
Record a fatal message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.fatal()
.- Parameters:
msg (str) – the message to be recorded
args – list of arguments
kw – list of keyword arguments
- flushOutput()
Flushes the log output
- getAttrDict()
- getChildren()
Returns the log children for this object
- Returns:
the list of log children
- Return type:
sequence<logging.Logger
- classmethod getLogFormat()
Retuns the current log message format (the root log format)
- Returns:
the log message format
- Return type:
- getLogFullName()
Gets the full log name for this object
- Returns:
the full log name
- Return type:
- classmethod getLogLevel()
Retuns the current log level (the root log level)
- Returns:
a number representing the log level
- Return type:
- getLogObj()
Returns the log object for this object
- Returns:
the log object
- Return type:
- classmethod getLogger(name=None)
- getParent()
Returns the log parent for this object or None if no parent exists
- Returns:
the log parent for this object
- Return type:
logging.Logger or None
- classmethod getRootLog()
Retuns the root logger
- Returns:
the root logger
- Return type:
- info(msg, *args, **kw)
Record an info message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.info()
.- Parameters:
msg (str) – the message to be recorded
args – list of arguments
kw – list of keyword arguments
- classmethod initRoot()
Class method to initialize the root logger. Do NOT call this method directly in your code
- log(level, msg, *args, **kw)
Record a log message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.log()
.
- log_format = <logging.Formatter object>
Default log message format
- log_level = 20
Current global log level
- removeLogHandler(handler)
Removes the given handler from this object’s logger
- Parameters:
handler (logging.Handler) – the handler to be removed
- classmethod removeRootLogHandler(h)
Removes the given handler from the root logger
- Parameters:
h (logging.Handler) – the handler to be removed
- classmethod resetLogFormat()
Resets the log message format (the root log format)
- classmethod resetLogLevel()
Resets the log level (the root log level)
- root_init_lock = <unlocked _thread.lock object>
Internal usage
- root_inited = True
Internal usage
- classmethod setLogFormat(format)
sets the new log message format
- Parameters:
format (str) – the new log message format
- classmethod setLogLevel(level)
sets the new log level (the root log level)
- Parameters:
level (int) – the new log level
- stack(target=5)
Log the usual stack information, followed by a listing of all the local variables in each frame.
- stream_handler = <StreamHandler <stderr> (NOTSET)>
the main stream handler
- syncLog()
Synchronises the log output
- trace(msg, *args, **kw)
Record a trace message in this object’s logger. Accepted args and kwargs are the same as
logging.Logger.log()
.- Parameters:
msg (str) – the message to be recorded
args – list of arguments
kw – list of keyword arguments
- traceback(level=5, extended=True)
Log the usual traceback information, followed by a listing of all the local variables in each frame.
- updateAttrDict(other)
- warning(msg, *args, **kw)
Record a warning message in this object’s logger. Accepted args and kwargs* are the same as
logging.Logger.warning()
.- Parameters:
msg (str) – the message to be recorded
args – list of arguments
kw – list of keyword arguments