SardanaDevice
Generic Sardana Tango device module
Classes
SardanaDevice
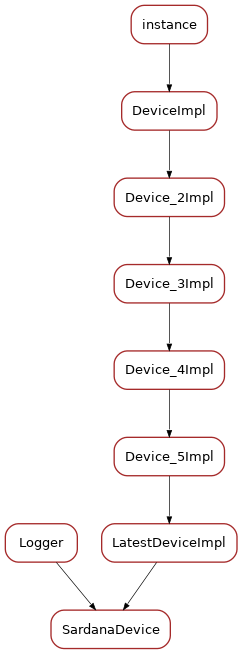
- class SardanaDevice(dclass, name)[source]
Bases:
LatestDeviceImpl
,Logger
SardanaDevice represents the base class for all Sardana
PyTango.DeviceImpl
classes- init(name)[source]
initialize the device once in the object lifetime. Override when necessary but always call the method from your super class
- Parameters:
name (
str
) – device name
- get_full_name()[source]
Compose full name from the TANGO_HOST information and device name.
Full name is of format “tango://dbhost:dbport/<domain>/<family>/<member>” where dbhost is always FQDN.
- Return type:
- Returns:
this device full name
- init_device()[source]
Initialize the device. Called during startup after
init()
and every time the tangoInit
command is executed. Override when necessary but always call the method from your super class
- sardana_init_hook()[source]
Hook that is called before the server event loop.
The idea behind this hook is to be equivalent to server_init_hook from Tango. Similar behaviour can be archived using post_init_callback.
- init_device_nodb()[source]
Internal method. Initialize the device when tango database is not being used (example: in demos)
- delete_device()[source]
Clean the device. Called during shutdown and every time the tango
Init
command is executed. Override when necessary but always call the method from your super class
- set_change_events(evts_checked, evts_not_checked)[source]
Helper method to set change events on attributes
- Parameters:
evts_checked (
Sequence
[str
]) – list of attribute names to activate change events programatically with tango filter activeevts_not_checked (
Sequence
[str
]) – list of attribute names to activate change events programatically with tango filter inactive. Use this with care! Attributes configured with no change event filter may potentially generated a lot of events!
- initialize_dynamic_attributes()[source]
Initialize dynamic attributes. Default implementation does nothing. Override when necessary.
- initialize_attribute_values()[source]
Initialize attributes values. Default implementation does nothing. Override when necessary.
- get_event_thread_pool()[source]
Return the
ThreadPool
used by sardana to send tango events.- Return type:
- Returns:
the sardana
ThreadPool
- get_attribute_by_name(attr_name)[source]
Gets the attribute for the given name.
- Parameters:
attr_name (
str
) – attribute name- Return type:
Attribute
- Returns:
the attribute object
- get_wattribute_by_name(attr_name)[source]
Gets the writable attribute for the given name.
- Parameters:
attr_name (
str
) – attribute name- Return type:
WAttribute
- Returns:
the attribute object
- get_database()[source]
Helper method to return a reference to the current tango database
- Return type:
Database
- Returns:
the Tango database
- set_attribute(attr, value=None, w_value=None, timestamp=None, quality=None, error=None, priority=1, synch=True)[source]
Sets the given attribute value. If timestamp is not given, now is used as timestamp. If quality is not given VALID is assigned. If error is given an error event is sent (with no value and quality INVALID). If priority is > 1, the event filter is temporarily disabled so the event is sent for sure. If synch is set to True, wait for fire event to finish
- Parameters:
attr (
Attribute
) – the tango attributevalue (
Optional
[Any
]) – the value to be set (not mandatory if setting an error) [default: None]w_value (
Optional
[Any
]) – the write value to be set (not mandatory) [default: None, meaning maintain current write value]timestamp (
Union
[float
,TimeVal
,None
]) – the timestamp associated with the operation [default: None, meaning use now as timestamp]quality (
Optional
[AttrQuality
]) – attribute quality [default: None, meaning VALID]error (
Optional
[DevFailed
]) – a tango DevFailed error or None if not an error [default: None]priority (
int
) – event priority [default: 1, meaning normal priority]. If priority is > 1, the event filter is temporarily disabled so the event is sent for sure. The event filter is restored to the previous valuesynch (
bool
) – If synch is set to True, wait for fire event to finish. If False, a job is sent to the sardana thread pool and the method returns immediately [default: True]
- Return type:
- set_attribute_push(attr, value=None, w_value=None, timestamp=None, quality=None, error=None, priority=1, synch=True)[source]
Synchronous internal implementation of
set_attribute()
(synch is passed to this method because it might need to know if it is being executed in a synchronous or asynchronous context).
- calculate_tango_state(ctrl_state, update=False)[source]
Calculate tango state based on the controller state.
- Parameters:
ctrl_state (
State
) – the state returned by the controllerupdate (
bool
) – if True, set the state of this device with the calculated tango state [default: False:
- Return type:
DevState
- Returns:
the corresponding tango state
SardanaDeviceClass
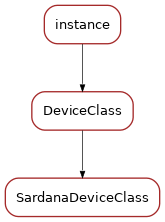
- class SardanaDeviceClass(name)[source]
Bases:
DeviceClass
SardanaDeviceClass represents the base class for all Sardana
PyTango.DeviceClass
classes- class_property_list = {}
Sardana device class properties definition
- device_property_list = {}
Sardana device properties definition
- cmd_list = {}
Sardana device command definition
- attr_list = {}
Sardana device attribute definition
- write_class_property()[source]
Write class properties
ProjectTitle
,Description
,doc_url
,InheritedFrom
and__icon
- dyn_attr(dev_list)[source]
Invoked to create dynamic attributes for the given devices. Default implementation calls
SardanaDevice.initialize_dynamic_attributes()
for each device- Parameters:
dev_list (
DeviceImpl
) – list of devices- Return type: