Taurus Extensions
Functions
Classes
BaseElement
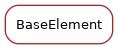
- class BaseElement[source]
Bases:
object
The base class for elements in the Pool (Pool itself, Motor, ControllerClass, ExpChannel all should inherit from this class directly or indirectly)
Controller
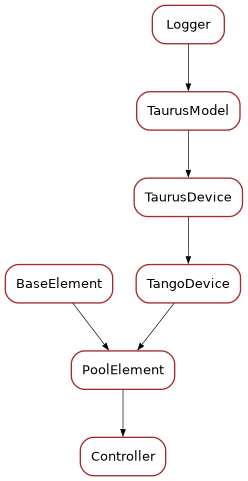
- class Controller(name, **kw)[source]
Bases:
PoolElement
Class encapsulating Controller functionality.
ControllerClass
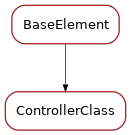
CTExpChannel
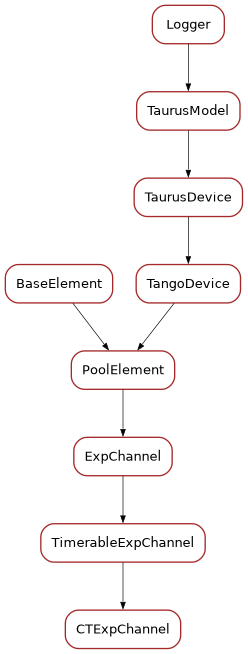
ExpChannel
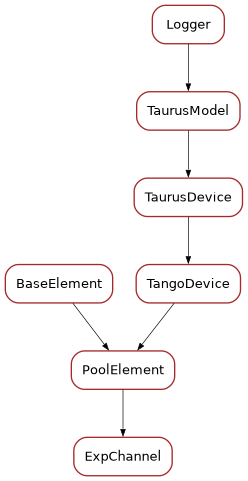
- class ExpChannel(name, **kw)[source]
Bases:
PoolElement
Class encapsulating ExpChannel functionality.
- getValueObj_()[source]
Retrurns Value attribute event generator object.
- Return type:
TangoAttributeEG
- Returns:
Value attribute event generator
..todo:: When support to Taurus 3 will be dropped provide getValueObj. Taurus 3 TaurusDevice class already uses this name.
- getValueRefObj()[source]
Return ValueRef attribute event generator object.
- Return type:
TangoAttributeEG
- Returns:
ValueRef attribute event generator
- go(*args, **kwargs)[source]
Count and report count result.
Configuration measurement, then start and wait until finish.
Note
The count (go) method API is partially experimental (value references may be changed to values whenever possible in the future). Backwards incompatible changes may occur if deemed necessary by the core developers.
- Return type:
- Returns:
state and value (or value reference - experimental)
- startCount(**kwargs)
- waitCount(timeout=None, id=None)
Wait for the operation to finish
- count(*args, **kwargs)
Count and report count result.
Configuration measurement, then start and wait until finish.
Note
The count (go) method API is partially experimental (value references may be changed to values whenever possible in the future). Backwards incompatible changes may occur if deemed necessary by the core developers.
- Return type:
- Returns:
state and value (or value reference - experimental)
- stopCount(synch=True, timeout=None, **kwargs)
Executes the “Abort” command on the element
- stop(synch=True, timeout=None, **kwargs)
Executes the “Stop” command on the element
- Parameters:
synch – If True, waits the Stop process to finish.
timeout – Wait timeout (in seconds). Only used if synch=True.
Instrument
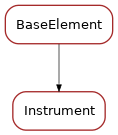
IORegister
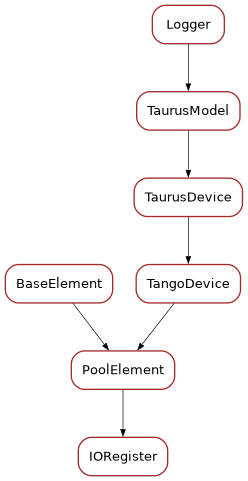
- class IORegister(name, **kw)[source]
Bases:
PoolElement
Class encapsulating IORegister functionality.
- getValueObj()[source]
- Deprecated by TEP14.
- ..warning::
this bck-compat implementation is not perfect because the rvalue of the returned TangoAttributeValue is now a member of TaurusDevState instead of TaurusSWDevState
Deprecated since version 4.0: Use state [agnostic] or stateObj.read [Tango] instead
- writeIORegister(new_value, timeout=None)
- writeIOR(new_value, timeout=None)
- readIORegister(force=False)
- readIOR(force=False)
- getValue(force=False)
MeasurementGroup
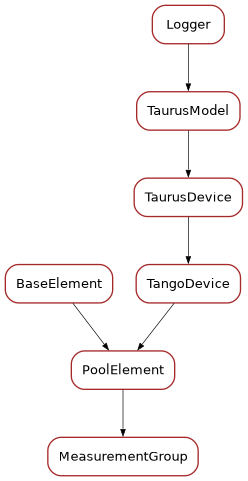
- class MeasurementGroup(name, **kw)[source]
Bases:
PoolElement
MeasurementGroup Sardana-Taurus extension.
Setting configuration parameters using e.g.,
setEnabled
orsetTimer
, etc. by default applies changes on the server. Since setting the configuration means passing to the server all the configuration parameters of the measurement group at once this behavior can be changed with theapply=False
. Then the configuration changes are kept locally. This is useful when changing more then one parameter. In this case only setting of the last parameter should useapply=True
or useapplyConfiguration
afterwards:# or in a macro use: meas_grp = self.getMeasurementGroup("mntgrp01") meas_grp = taurus.Device("mntgrp01") meas_grp.setEnabled(False, apply=False) meas_grp.setEnabled(True, "ct01", "ct02")
- setOutput(output, *elements, apply=True)[source]
Set the output configuration for the given elements.
Channels and controllers are accepted as elements. Setting the output on the controller means setting it to all channels of this controller present in this measurement group.
- getOutput(*elements, ret_full_name=False)[source]
Get the output configuration of the given elements.
Channels and controllers are accepted as elements. Getting the output from the controller means getting it from all channels of this controller present in this measurement group.
- Parameters:
- Return type:
- Returns:
ordered dictionary where keys are channel names (or full names if
ret_full_name=True
) and values are their output configurations. Note that even if the elements contained controllers, the returned configuration will always contain only channels.
- setEnabled(enabled, *elements, apply=True)[source]
Set the enabled configuration for the given elements.
Channels and controllers are accepted as elements. Setting the enabled on the controller means setting it to all channels of this controller present in this measurement group.
- getEnabled(*elements, ret_full_name=False)[source]
Get the output configuration of the given elements.
Channels and controllers are accepted as elements. Getting the enabled from the controller means getting it from all channels of this controller present in this measurement group.
- Parameters:
- Return type:
- Returns:
ordered dictionary where keys are channel names (or full names if
ret_full_name=True
) and values are their output configurations. Note that even if the elements contained controllers, the returned configuration will always contain only channels.
- setPlotType(plot_type, *elements, apply=True)[source]
Set the enabled configuration for the given elements.
Channels and controllers are accepted as elements. Setting the plot type on the controller means setting it to all channels of this controller present in this measurement group.
- getPlotType(*elements, ret_full_name=False)[source]
Get the output configuration of the given elements.
Channels and controllers are accepted as elements. Getting the plot type from the controller means getting it from all channels of this controller present in this measurement group.
- Parameters:
- Return type:
- Returns:
ordered dictionary where keys are channel names (or full names if
ret_full_name=True
) and values are their output configurations. Note that even if the elements contained controllers, the returned configuration will always contain only channels.
- setPlotAxes(plot_axes, *elements, apply=True)[source]
Set the enabled configuration for the given elements.
Channels and controllers are accepted as elements. Setting the plot axes on the controller means setting it to all channels of this controller present in this measurement group.
- getPlotAxes(*elements, ret_full_name=False)[source]
Get the output configuration of the given elements.
Channels and controllers are accepted as elements. Getting the plot axes from the controller means getting it from all channels of this controller present in this measurement group.
- Parameters:
- Return type:
- Returns:
ordered dictionary where keys are channel names (or full names if
ret_full_name=True
) and values are their output configurations. Note that even if the elements contained controllers, the returned configuration will always contain only channels.
- setValueRefEnabled(value_ref_enabled, *elements, apply=True)[source]
Set the output configuration for the given elements.
Channels and controllers are accepted as elements. Setting the value reference enabled on the controller means setting it to all channels of this controller present in this measurement group.
- getValueRefEnabled(*elements, ret_full_name=False)[source]
Get the value reference enabled configuration of the given elements.
Channels and controllers are accepted as elements. Getting the value from the controller means getting it from all channels of this controller present in this measurement group.
- Parameters:
- Return type:
- Returns:
ordered dictionary where keys are channel names (or full names if
ret_full_name=True
) and values are their output configurations. Note that even if the elements contained controllers, the returned configuration will always contain only channels.
- setValueRefPattern(value_ref_pattern, *elements, apply=True)[source]
Set the output configuration for the given elements.
Channels and controllers are accepted as elements. Setting the value reference pattern on the controller means setting it to all channels of this controller present in this measurement group.
- getValueRefPattern(*elements, ret_full_name=False)[source]
Get the value reference enabled configuration of the given elements.
Channels and controllers are accepted as elements. Getting the value from the controller means getting it from all channels of this controller present in this measurement group.
- Parameters:
- Return type:
- Returns:
ordered dictionary where keys are channel names (or full names if
ret_full_name=True
) and values are their output configurations. Note that even if the elements contained controllers, the returned configuration will always contain only channels.
- setTimer(timer, *elements, apply=True)[source]
Set the timer configuration for the given channels of the same controller.
Note
Currently the controller’s timer must be unique. Hence this method will set it for the whole controller regardless of the
elements
argument.
- getTimer(*elements, ret_full_name=False, ret_by_ctrl=False)[source]
Get the timer configuration of the given elements.
Channels and controllers are accepted as elements. Getting the output from the controller means getting it from all channels of this controller present in this measurement group, unless
ret_by_ctrl=True
.- Parameters:
elements (
str
) – sequence of element names or full names, no elements means get from allret_full_name (
bool
) – whether keys in the returned dictionary are full names or names (default:False
means return names)ret_by_ctrl (
bool
) – whether keys in the returned dictionary are controllers or channels (default:False
means return channels)
- Return type:
- Returns:
ordered dictionary where keys are channel names (or full names if
ret_full_name=True
) and values are their timer configurations
- setMonitor(monitor, *elements, apply=True)[source]
Set the monitor configuration for the given channels of the same controller.
Note
Currently the controller’s monitor must be unique. Hence this method will set it for the whole controller regardless of the
elements
argument.
- getMonitor(*elements, ret_full_name=False, ret_by_ctrl=False)[source]
Get the monitor configuration of the given elements.
Channels and controllers are accepted as elements. Getting the output from the controller means getting it from all channels of this controller present in this measurement group, unless
ret_by_ctrl=True
.- Parameters:
elements (
str
) – sequence of element names or full names, no elements means get from allret_full_name (
bool
) – whether keys in the returned dictionary are full names or names (default:False
means return names)ret_by_ctrl (
bool
) – whether keys in the returned dictionary are controllers or channels (default:False
means return channels)
- Return type:
- Returns:
ordered dictionary where keys are channel names (or full names if
ret_full_name=True
) and values are their monitor configurations
- setSynchronizer(synchronizer, *elements, apply=True)[source]
Set the synchronizer configuration for the given channels or controller.
Note
Currently the controller’s synchronizer must be unique. Hence this method will set it for the whole controller regardless of the
elements
argument.
- getSynchronizer(*elements, ret_full_name=False, ret_by_ctrl=False)[source]
Get the synchronizer configuration of the given elements.
Channels and controllers are accepted as elements. Getting the output from the controller means getting it from all channels of this controller present in this measurement group, unless
ret_by_ctrl=True
.- Parameters:
elements (
str
) – sequence of element names or full names, no elements means get from allret_full_name (
bool
) – whether keys in the returned dictionary are full names or names (default:False
means return names)ret_by_ctrl (
bool
) – whether keys in the returned dictionary are controllers or channels (default:False
means return channels)
- Return type:
- Returns:
ordered dictionary where keys are channel names (or full names if
ret_full_name=True
) and values are their synchronizer configurations
- setSynchronization(synchronization, *elements, apply=True)[source]
Set the synchronization configuration for the given channels or controller.
Note
Currently the controller’s synchronization must be unique. Hence this method will set it for the whole controller regardless of the
elements
argument.- Parameters:
- Return type:
- getSynchronization(*elements, ret_full_name=False, ret_by_ctrl=False)[source]
Get the synchronization configuration of the given elements.
Channels and controllers are accepted as elements. Getting the output from the controller means getting it from all channels of this controller present in this measurement group, unless
ret_by_ctrl=True
.- Parameters:
elements (
str
) – sequence of element names or full names, no elements means get from allret_full_name (
bool
) – whether keys in the returned dictionary are full names or names (default:False
means return names)ret_by_ctrl (
bool
) – whether keys in the returned dictionary are controllers or channels (default:False
means return channels)
- Return type:
- Returns:
ordered dictionary where keys are channel names (or full names if
ret_full_name=True
) and values are their synchronization configurations
- applyConfiguration()[source]
Apply configuration changes kept locally on the server.
Setting configuration parameters using e.g.,
setEnabled
orsetTimer
, etc. withapply=False
keeps the changes locally. Use this method to apply them on the server afterwards.
- getChannelsEnabledInfo()[source]
Returns information about only enabled channels present in the measurement group in a form of ordered, based on the channel index, list. :rtype:
List
[TangoChannelInfo
] :return: list with channels info
- enableChannels(channels)[source]
DEPRECATED: Enable acquisition of the indicated channels.
- Parameters:
channels – (seq<str>) a sequence of strings indicating channel names
- disableChannels(channels)[source]
DEPRECATED: Disable acquisition of the indicated channels.
- Parameters:
channels – (seq<str>) a sequence of strings indicating channel names
- valueBufferChanged(channel, value_buffer)[source]
Receive value buffer updates, pre-process them, and call the subscribed callback.
- Parameters:
channel (
ExpChannel
) – channel that reports value buffer updatevalue_buffer (
str
) – json encoded value buffer update, it contains at least values and indexes
- Return type:
- subscribeValueBuffer(cb=None)[source]
Subscribe to channels’ value buffer update events. If no callback is passed, the default channel’s callback is subscribed which will store the data in the channel’s value_buffer attribute.
- unsubscribeValueBuffer(cb=None)[source]
Unsubscribe from channels’ value buffer events. If no callback is passed, unsubscribe the channel’s default callback.
- valueRefBufferChanged(channel, value_ref_buffer)[source]
Receive value ref buffer updates, pre-process them, and call the subscribed callback.
- Parameters:
channel (
ExpChannel
) – channel that reports value ref buffer updatevalue_ref_buffer (
str
) – json encoded value ref buffer update, it contains at least value refs and indexes
- Return type:
- subscribeValueRefBuffer(cb=None)[source]
Subscribe to channels’ value ref buffer update events. If no callback is passed, the default channel’s callback is subscribed which will store the data in the channel’s value_buffer attribute.
- unsubscribeValueRefBuffer(cb=None)[source]
Unsubscribe from channels’ value ref buffer events. If no callback is passed, unsubscribe the channel’s default callback.
- count_raw(start_time=None)[source]
Raw count and report count values.
Simply start and wait until finish, no configuration nor preparation.
Note
The count_raw method API is partially experimental (value references may be changed to values whenever possible in the future). Backwards incompatible changes may occur if deemed necessary by the core developers.
- go(*args, **kwargs)[source]
Count and report count values.
Configuration and prepare for measurement, then start and wait until finish.
Note
The count (go) method API is partially experimental (value references may be changed to values whenever possible in the future). Backwards incompatible changes may occur if deemed necessary by the core developers.
- count_continuous(synch_description, value_buffer_cb=None, value_ref_buffer_cb=None)[source]
Execute measurement process according to the given synchronization description.
- Parameters:
- Return type:
- Returns:
state and eventually value buffers if no callback was passed
Todo
Think of unifying measure with count.
Note
The measure method has been included in MeasurementGroup class on a provisional basis. Backwards incompatible changes (up to and including removal of the method) may occur if deemed necessary by the core developers.
- startCount(**kwargs)
- waitCount(timeout=None, id=None)
Wait for the operation to finish
- count(*args, **kwargs)
Count and report count values.
Configuration and prepare for measurement, then start and wait until finish.
Note
The count (go) method API is partially experimental (value references may be changed to values whenever possible in the future). Backwards incompatible changes may occur if deemed necessary by the core developers.
- stopCount(synch=True, timeout=None, **kwargs)
Executes the “Abort” command on the element
- stop(synch=True, timeout=None, **kwargs)
Executes the “Stop” command on the element
- Parameters:
synch – If True, waits the Stop process to finish.
timeout – Wait timeout (in seconds). Only used if synch=True.
Motor
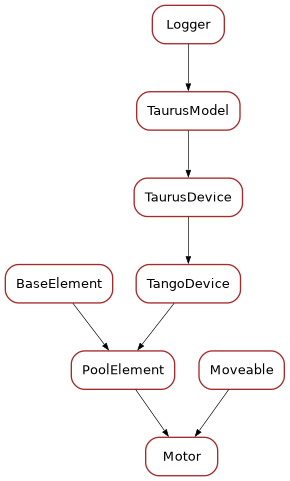
- class Motor(name, **kw)[source]
Bases:
PoolElement
,Moveable
Class encapsulating Motor functionality.
- startMove(sequence<float> new_pos, double timeout=None) sequence<id>
Calling this method will trigger a movement off all components of the movement.
- waitMove(timeout=None, id=None)
Wait for the operation to finish
- getLastMotionTime()
Returns the time it took for last go operation
- getTotalLastMotionTime()
Returns the time it took for last go operation, including dead time to prepare, wait for events, etc
MotorGroup
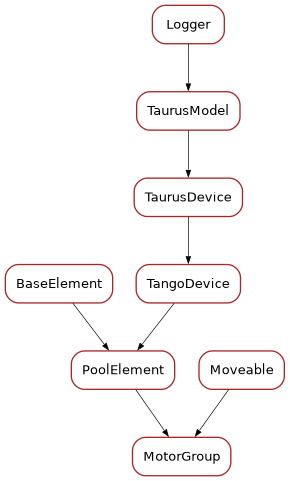
- class MotorGroup(name, **kw)[source]
Bases:
PoolElement
,Moveable
Class encapsulating MotorGroup functionality.
- startMove(sequence<float> new_pos, double timeout=None) sequence<id>
Calling this method will trigger a movement off all components of the movement.
- waitMove(timeout=None, id=None)
Wait for the operation to finish
- getLastMotionTime()
Returns the time it took for last go operation
- getTotalLastMotionTime()
Returns the time it took for last go operation, including dead time to prepare, wait for events, etc
OneDExpChannel
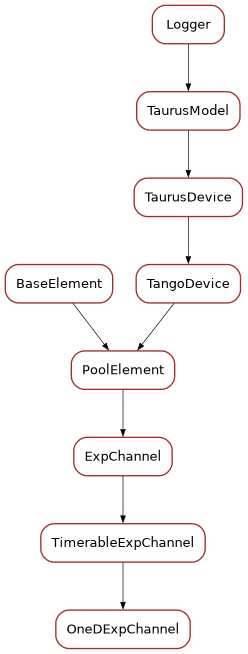
Pool
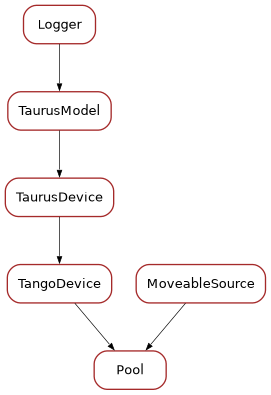
- class Pool(name, **kw)[source]
Bases:
TangoDevice
,MoveableSource
Class encapsulating device Pool functionality.
- reconfigObj(obj)[source]
Reconfigure (initialize)
obj
in the server- This includes:
recreation of the device and its attributes (Tango)
writing of the memorized attributes (last set values)
Usefull when there was a problem with the hardware.
Note
If
obj
is aController
this will usually also require to call it with the controller’s elements.
PoolElement
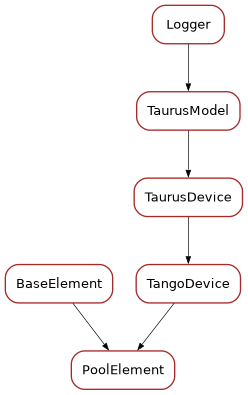
- class PoolElement(name, **kwargs)[source]
Bases:
BaseElement
,TangoDevice
Base class for a Pool element device.
- reconfig()[source]
Reconfigure (initialize) element in the server
- This includes:
recreation of the device and its attributes (Tango)
writing of the memorized attributes (last set values)
Usefull when there was a problem with the hardware.
Note
Calling this method on the
Controller
will usually also require to call it on the controller’s elements.
- getStatusEG()[source]
Get Status attribute event generator.
First call to this method will create the attribute event generator object and store it for future references. Further calls will return the same object.
- Return type:
- getTotalLastGoTime()[source]
Returns the time it took for last go operation, including dead time to prepare, wait for events, etc
- stop(synch=True, timeout=None, **kwargs)[source]
Executes the “Stop” command on the element
- Parameters:
synch – If True, waits the Stop process to finish.
timeout – Wait timeout (in seconds). Only used if synch=True.
PseudoCounter
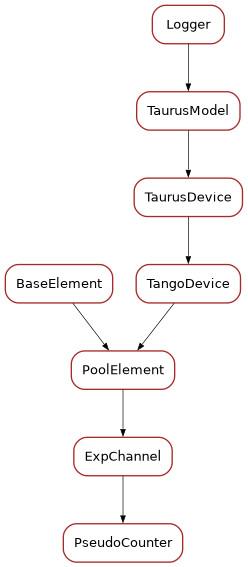
- class PseudoCounter(name, **kw)[source]
Bases:
ExpChannel
Class encapsulating PseudoCounter functionality.
PseudoMotor
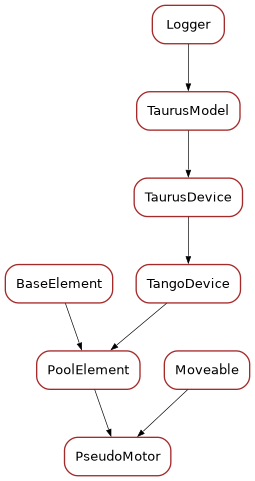
- class PseudoMotor(name, **kw)[source]
Bases:
PoolElement
,Moveable
Class encapsulating PseudoMotor functionality.
- startMove(sequence<float> new_pos, double timeout=None) sequence<id>
Calling this method will trigger a movement off all components of the movement.
- waitMove(timeout=None, id=None)
Wait for the operation to finish
- getLastMotionTime()
Returns the time it took for last go operation
- getTotalLastMotionTime()
Returns the time it took for last go operation, including dead time to prepare, wait for events, etc
TriggerGate
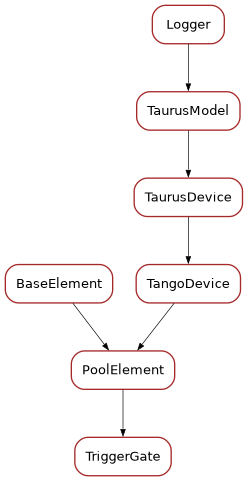
- class TriggerGate(name, **kwargs)[source]
Bases:
PoolElement
Class encapsulating TriggerGate functionality.
TwoDExpChannel
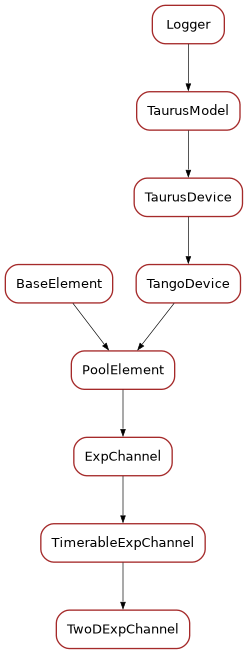
ZeroDExpChannel
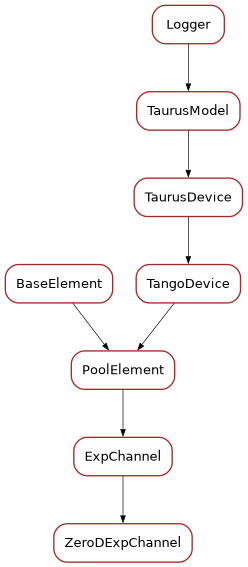
- class ZeroDExpChannel(name, **kw)[source]
Bases:
ExpChannel
Class encapsulating ZeroDExpChannel functionality.