poolaction
This module is part of the Python Pool libray. It defines the class for an abstract action over a set of pool elements
Functions
Classes
- get_thread_pool()[source]
Returns the global pool of threads for Sardana
- Return type:
- Returns:
the global pool of threads object
PoolAction
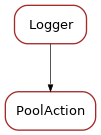
- class PoolAction(main_element, name='GlobalAction')[source]
Bases:
Logger
A generic class to handle any type of operation (like motion or acquisition)
- OperationContextClass
alias of
OperationContext
- get_main_element()[source]
Returns the main element for this action
- Returns:
sardana.pool.poolelement.PoolElement
- property main_element
Returns the main element for this action
- Returns:
sardana.pool.poolelement.PoolElement
- property pool
Returns the pool object for this action
- Returns:
sardana.pool.pool.Pool
- add_element(element)[source]
Adds a new element to this action.
- Parameters:
element (
PoolElement
) – the new element to be added- Return type:
- remove_element(element)[source]
Removes an element from this action. If the element is not part of this action, a ValueError is raised.
- Parameters:
element (
PoolElement
) – the new element to be removed- Raises:
ValueError
- Return type:
- get_elements(copy_of=False)[source]
Returns a sequence of all elements involved in this action.
- Parameters:
copy_of (
bool
) – If False (default) the internal container of elements is returned. If True, a copy of the internal container is returned instead- Return type:
- Returns:
a sequence of all elements involved in this action.
- get_pool_controller_list()[source]
Returns a list of all controller elements involved in this action.
- Return type:
- Returns:
a list of all controller elements involved in this action.
- get_pool_controllers()[source]
Returns a dict of all controller elements involved in this action.
- Return type:
- Returns:
a dict of all controller elements involved in this action.
- property pool_controllers: Dict[PoolController, Sequence[PoolElement]]
Returns a dict of all controller elements involved in this action.
- Returns:
a dict of all controller elements involved in this action.
- is_running()[source]
Determines if this action is running or not
- Return type:
- Returns:
True if action is running or False otherwise
- start_action(*args, **kwargs)[source]
Start procedure for this action. Default implementation raises NotImplementedError
- Raises:
NotImplementedError
- set_finish_hooks(hooks)[source]
Set finish hooks for this action.
- Parameters:
hooks (
Optional
[OrderedDict
]) – an ordered dictionary where keys are the hooks and values is a flag if the hook is permanent (not removed after the execution)- Return type:
- finish_action()[source]
Finishes the action execution. If a finish hook is defined it safely executes it. Otherwise nothing happens
- was_stopped()[source]
Determines if the action has been stopped from outside
- Return type:
- Returns:
True if action has been stopped from outside or False otherwise
- was_aborted()[source]
Determines if the action has been aborted from outside
- Return type:
- Returns:
True if action has been aborted from outside or False otherwise
- was_released()[source]
Determines if the action has been released from outside
- Return type:
- Returns:
True if action has been released from outside or False otherwise
- was_action_interrupted()[source]
Determines if the action has been interruped from outside (either from an abort or a stop).
- Return type:
- Returns:
True if action has been interruped from outside or False otherwise
- action_loop()[source]
Action loop for this action. Default implementation raises NotImplementedError
- Raises:
NotImplementedError
- read_state_info(ret=None, serial=False)[source]
Reads state information of all elements involved in this action
- Parameters:
- Return type:
- Returns:
a map containing state information per element
- raw_read_state_info(ret=None, serial=False)[source]
Unsafe. Reads state information of all elements involved in this action
- Parameters:
- Return type:
- Returns:
a map containing state information per element
- read_value(ret=None, serial=False)[source]
Reads value information of all elements involved in this action
- Parameters:
- Return type:
- Returns:
a map containing value information per element (value object, Exception or None)>
- raw_read_value(ret=None, serial=False)[source]
Unsafe. Reads value information of all elements involved in this action
- Parameters:
- Return type:
- Returns:
a map containing value information per element
sardana.sardanavalue.SardanaValue
>
- read_value_loop(ret=None, serial=False)[source]
Reads value information of all elements involved in this action
- Parameters:
- Return type:
- Returns:
a map containing value information per element (value object, Exception or None)>
- raw_read_value_loop(ret=None, serial=False)[source]
Unsafe. Reads value information of all elements involved in this action
- Parameters:
- Return type:
- Returns:
a map containing value information per element
sardana.sardanavalue.SardanaValue
>
OperationInfo
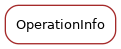
PoolActionItem
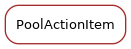
ActionContext
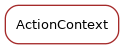