sardanaattribute
This module is part of the Python Sardana libray. It defines the base classes for Sardana attributes
Classes
SardanaAttribute
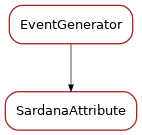
- class SardanaAttribute(obj, name=None, initial_value=None, **kwargs)[source]
Class representing an atomic attribute like position of a motor or a counter value
- has_value()[source]
Determines if the attribute’s read value has been read at least once in the lifetime of the attribute.
- Return type:
- Returns:
True if the attribute has a read value stored or False otherwise
- has_write_value()[source]
Determines if the attribute’s write value has been read at least once in the lifetime of the attribute.
- Return type:
- Returns:
True if the attribute has a write value stored or False otherwise
- get_obj()[source]
Returns the object which owns this attribute
- Return type:
- Returns:
the object which owns this attribute
- in_error()[source]
Determines if this attribute is in error state.
- Return type:
- Returns:
True if the attribute is in error state or False otherwise
- set_value(value, exc_info=None, timestamp=None, propagate=1)[source]
Sets the current read value and propagates the event (if propagate > 0).
- Parameters:
value (
Union
[Any
,SardanaValue
]) – the new read value for this attribute. If a SardanaValue is given, exc_info and timestamp are ignored (if given)exc_info (
Optional
[Tuple
]) – exception information as returned bysys.exc_info()
[default: None, meaning no exception]timestamp (
Optional
[float
]) – timestamp of attribute readout [default: None, meaning create a ‘now’ timestamp]propagate (
int
) – 0 for not propagating, 1 to propagate, 2 propagate with priority
- Return type:
- get_value_obj()[source]
Returns the last read value for this attribute.
- Return type:
- Returns:
the last read value for this attribute
- Raises:
Exception
if no read value has been set yet
- set_write_value(w_value, timestamp=None, propagate=1)[source]
Sets the current write value.
- Parameters:
- Return type:
- get_write_value()[source]
Returns the last write value for this attribute.
- Return type:
- Returns:
the last write value for this attribute or None if value has not been written yet
- get_write_value_obj()[source]
Returns the last write value object for this attribute.
- Return type:
- Returns:
the last write value for this attribute or None if value has not been written yet
- get_exc_info()[source]
Returns the exception information (like
sys.exc_info()
) about last attribute readout or None if last read did not generate an exception.
- get_timestamp()[source]
Returns the timestamp of the last readout or None if the attribute has never been read before
- get_write_timestamp()[source]
Returns the timestamp of the last write or None if the attribute has never been written before
- fire_write_event(propagate=1)[source]
Fires an event to the listeners of the object which owns this attribute.
- fire_read_event(propagate=1)[source]
Fires an event to the listeners of the object which owns this attribute.
- property obj: Any
Returns the object which owns this attribute
- Returns:
the object which owns this attribute
- property value_obj: SardanaValue
Returns the last read value for this attribute.
- Returns:
the last read value for this attribute
- Raises:
Exception
if no read value has been set yet
- property write_value_obj: SardanaValue
Returns the last write value object for this attribute.
- Returns:
the last write value for this attribute or None if value has not been written yet
- property value: Any
Returns the last read value for this attribute.
- Returns:
the last read value for this attribute
- Raises:
Exception
if no read value has been set yet
- property w_value: object
Returns the last write value for this attribute.
- Returns:
the last write value for this attribute or None if value has not been written yet
- property quality
- property error: bool
Determines if this attribute is in error state.
- Returns:
True if the attribute is in error state or False otherwise
- property exc_info: Tuple | None
Returns the exception information (like
sys.exc_info()
) about last attribute readout or None if last read did not generate an exception.- Returns:
exception information or None
- add_listener(listener)
Adds a new listener for this object.
- Parameters:
listener – a listener
- fire_event(event_type, event_value, listeners=None)
- flush_queue()
- get_listeners()
- has_listeners()
Returns True if anybody is listening to events from this object
- Returns:
True is at least one listener is listening or False otherwise
- queue_event(event_type, event_value, listeners=None)
- remove_listener(listener)
Removes an existing listener for this object.
- Parameters:
listener – the listener to be removed
- Returns:
True is succeeded or False otherwise
SardanaSoftwareAttribute
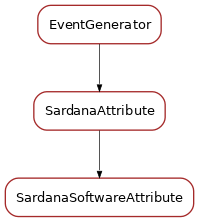
- class SardanaSoftwareAttribute(obj, name=None, initial_value=None, **kwargs)[source]
Class representing a software attribute. The difference between this and
SardanaAttribute
is that, because it is a pure software attribute, there is no difference ever between the read and write values.- get_value()
Returns the last read value for this attribute.
- set_value(value, exc_info=None, timestamp=None, propagate=1)[source]
Sets the current read value and propagates the event (if propagate > 0).
- Parameters:
value (
Any
) – the new read value for this attributeexc_info (
Optional
[Tuple
]) – exception information as returned bysys.exc_info()
[default: None, meaning no exception]timestamp (
Optional
[float
]) – timestamp of attribute readout [default: None, meaning create a ‘now’ timestamp]propagate (
int
) – 0 for not propagating, 1 to propagate, 2 propagate with priority
- Return type:
- property value: Any
Returns the last read value for this attribute.
- Returns:
the last read value for this attribute
- Raises:
Exception
if no read value has been set yet
- accepts(propagate)
- add_listener(listener)
Adds a new listener for this object.
- Parameters:
listener – a listener
- property error: bool
Determines if this attribute is in error state.
- Returns:
True if the attribute is in error state or False otherwise
- property exc_info: Tuple | None
Returns the exception information (like
sys.exc_info()
) about last attribute readout or None if last read did not generate an exception.- Returns:
exception information or None
- fire_event(event_type, event_value, listeners=None)
- fire_read_event(propagate=1)
Fires an event to the listeners of the object which owns this attribute.
- fire_write_event(propagate=1)
Fires an event to the listeners of the object which owns this attribute.
- flush_queue()
- get_exc_info()
Returns the exception information (like
sys.exc_info()
) about last attribute readout or None if last read did not generate an exception.
- get_listeners()
- get_obj()
Returns the object which owns this attribute
- Return type:
- Returns:
the object which owns this attribute
- get_quality()
- get_timestamp()
Returns the timestamp of the last readout or None if the attribute has never been read before
- get_value_obj()
Returns the last read value for this attribute.
- Return type:
- Returns:
the last read value for this attribute
- Raises:
Exception
if no read value has been set yet
- get_write_timestamp()
Returns the timestamp of the last write or None if the attribute has never been written before
- get_write_value()
Returns the last write value for this attribute.
- Return type:
- Returns:
the last write value for this attribute or None if value has not been written yet
- get_write_value_obj()
Returns the last write value object for this attribute.
- Return type:
- Returns:
the last write value for this attribute or None if value has not been written yet
- has_listeners()
Returns True if anybody is listening to events from this object
- Returns:
True is at least one listener is listening or False otherwise
- has_value()
Determines if the attribute’s read value has been read at least once in the lifetime of the attribute.
- Return type:
- Returns:
True if the attribute has a read value stored or False otherwise
- has_write_value()
Determines if the attribute’s write value has been read at least once in the lifetime of the attribute.
- Return type:
- Returns:
True if the attribute has a write value stored or False otherwise
- in_error()
Determines if this attribute is in error state.
- Return type:
- Returns:
True if the attribute is in error state or False otherwise
- property obj: Any
Returns the object which owns this attribute
- Returns:
the object which owns this attribute
- property quality
- queue_event(event_type, event_value, listeners=None)
- remove_listener(listener)
Removes an existing listener for this object.
- Parameters:
listener – the listener to be removed
- Returns:
True is succeeded or False otherwise
- set_quality(quality)
- set_write_value(w_value, timestamp=None, propagate=1)
Sets the current write value.
- Parameters:
- Return type:
- property value_obj: SardanaValue
Returns the last read value for this attribute.
- Returns:
the last read value for this attribute
- Raises:
Exception
if no read value has been set yet
- property w_value: object
Returns the last write value for this attribute.
- Returns:
the last write value for this attribute or None if value has not been written yet
- property write_value_obj: SardanaValue
Returns the last write value object for this attribute.
- Returns:
the last write value for this attribute or None if value has not been written yet
ScalarNumberAttribute
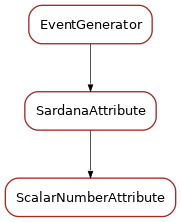
- class ScalarNumberAttribute(*args, **kwargs)[source]
A
SardanaAttribute
specialized for numbers- accepts(propagate)
- add_listener(listener)
Adds a new listener for this object.
- Parameters:
listener – a listener
- property error: bool
Determines if this attribute is in error state.
- Returns:
True if the attribute is in error state or False otherwise
- property exc_info: Tuple | None
Returns the exception information (like
sys.exc_info()
) about last attribute readout or None if last read did not generate an exception.- Returns:
exception information or None
- fire_event(event_type, event_value, listeners=None)
- fire_read_event(propagate=1)
Fires an event to the listeners of the object which owns this attribute.
- fire_write_event(propagate=1)
Fires an event to the listeners of the object which owns this attribute.
- flush_queue()
- get_exc_info()
Returns the exception information (like
sys.exc_info()
) about last attribute readout or None if last read did not generate an exception.
- get_listeners()
- get_obj()
Returns the object which owns this attribute
- Return type:
- Returns:
the object which owns this attribute
- get_quality()
- get_timestamp()
Returns the timestamp of the last readout or None if the attribute has never been read before
- get_value()
Returns the last read value for this attribute.
- get_value_obj()
Returns the last read value for this attribute.
- Return type:
- Returns:
the last read value for this attribute
- Raises:
Exception
if no read value has been set yet
- get_write_timestamp()
Returns the timestamp of the last write or None if the attribute has never been written before
- get_write_value()
Returns the last write value for this attribute.
- Return type:
- Returns:
the last write value for this attribute or None if value has not been written yet
- get_write_value_obj()
Returns the last write value object for this attribute.
- Return type:
- Returns:
the last write value for this attribute or None if value has not been written yet
- has_listeners()
Returns True if anybody is listening to events from this object
- Returns:
True is at least one listener is listening or False otherwise
- has_value()
Determines if the attribute’s read value has been read at least once in the lifetime of the attribute.
- Return type:
- Returns:
True if the attribute has a read value stored or False otherwise
- has_write_value()
Determines if the attribute’s write value has been read at least once in the lifetime of the attribute.
- Return type:
- Returns:
True if the attribute has a write value stored or False otherwise
- in_error()
Determines if this attribute is in error state.
- Return type:
- Returns:
True if the attribute is in error state or False otherwise
- property obj: Any
Returns the object which owns this attribute
- Returns:
the object which owns this attribute
- property quality
- queue_event(event_type, event_value, listeners=None)
- remove_listener(listener)
Removes an existing listener for this object.
- Parameters:
listener – the listener to be removed
- Returns:
True is succeeded or False otherwise
- set_quality(quality)
- set_value(value, exc_info=None, timestamp=None, propagate=1)
Sets the current read value and propagates the event (if propagate > 0).
- Parameters:
value (
Union
[Any
,SardanaValue
]) – the new read value for this attribute. If a SardanaValue is given, exc_info and timestamp are ignored (if given)exc_info (
Optional
[Tuple
]) – exception information as returned bysys.exc_info()
[default: None, meaning no exception]timestamp (
Optional
[float
]) – timestamp of attribute readout [default: None, meaning create a ‘now’ timestamp]propagate (
int
) – 0 for not propagating, 1 to propagate, 2 propagate with priority
- Return type:
- set_write_value(w_value, timestamp=None, propagate=1)
Sets the current write value.
- Parameters:
- Return type:
- property value: Any
Returns the last read value for this attribute.
- Returns:
the last read value for this attribute
- Raises:
Exception
if no read value has been set yet
- property value_obj: SardanaValue
Returns the last read value for this attribute.
- Returns:
the last read value for this attribute
- Raises:
Exception
if no read value has been set yet
- property w_value: object
Returns the last write value for this attribute.
- Returns:
the last write value for this attribute or None if value has not been written yet
- property write_value_obj: SardanaValue
Returns the last write value object for this attribute.
- Returns:
the last write value for this attribute or None if value has not been written yet
SardanaAttributeConfiguration
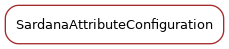
- class SardanaAttributeConfiguration[source]
Storage class for
SardanaAttribute
information (like ranges)- NoRange = (-inf, inf)