scan
This is the macro server scan module
Modules
Classes
GScan
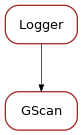
- class GScan(macro, generator=None, moveables=[], env={}, constraints=[], extrainfodesc=[])[source]
Bases:
Logger
Generic Scan object. The idea is that the scan macros create an instance of this Generic Scan, supplying in the constructor a reference to the macro that created the scan, a generator function pointer, a list of moveable items, an extra environment and a sequence of constrains.
If the referenced macro is hookable,
pre-scan
andpost-scan
hook hints will be used to execute callables before the start and after the end of the scan, respectivelyThe generator must be a function yielding a dictionary with the following content (minimum) at each step of the scan:
‘positions’ : In a step scan, a sequence with position(s) where the moveable(s) should go.
None
orfloat("NaN")
in the sequence means do not move a given moveable.‘integ_time’ : In a step scan, a number representing the integration time for the step (in seconds)
‘integ_time’ : In a continuous scan, the time between acquisitions
‘pre-move-hooks’ : (optional) a sequence of callables to be called in strict order before starting to move.
‘post-move-hooks’: (optional) a sequence of callables to be called in strict order after finishing the move.
‘pre-acq-hooks’ : (optional) a sequence of callables to be called in strict order before starting to acquire.
‘post-acq-hooks’ : (optional) a sequence of callables to be called in strict order after finishing acquisition but before recording the step.
‘post-step-hooks’ : (optional) a sequence of callables to be called in strict order after finishing recording the step.
‘point_id’ : a hashable identifing the scan point.
‘check_func’ : (optional) a list of callable objects. callable(moveables, counters)
‘extravalues’: (optional) a dictionary containing the values for each extra info field. The extra information fields must be described in extradesc (passed in the constructor of the Gscan)
Generator may accept a
bool
kwargestimate
which will be set toTrue
when the generator will be iterated to estimate the scan time.The moveables must be a sequence Motion or MoveableDesc objects.
The environment is a dictionary of extra environment to be added specific to the macro in question.
Each constrain must be a callable which must receive a two parameters: the current point and the next point. It should return True or False
The extradesc optional argument consists of a list of ColumnDesc objects which describe the data fields that will be filled using step[‘extravalues’], where step is what the generator yields.
The Generic Scan will create:
a ScanData
DataHandler with the following recorders:
OutputRecorder (depends on
OutputCols
environment variable)SharedMemoryRecorder (depends on
SharedMemory
environment variable)FileRecorder (depends on
ScanDir
,ScanData
andScanRecorder
environment variables)ScanDataEnvironment with the following contents:
‘serialno’ : a integer identifier for the scan operation
‘user’ : the user which started the scan
‘title’ : the scan title (build from macro.getMacroCommand)
‘datadesc’ : a seq<ColumnDesc> describing each column of data (labels, data format, data shape, etc)
‘estimatedtime’ : a float representing an estimation for the duration of the scan (in seconds). Negative means the time estimation is known not to be accurate. Anyway, time estimation has ‘at least’ semantics.
‘total_scan_intervals’ : total number of scan intervals. Negative means the estimation is known not to be accurate. In this case, estimation has ‘at least’ semantics.
‘’ : a datetime.datetime representing the start of the scan
‘instrumentlist’ : a list of Instrument objects containing info about the physical setup of the motors, counters,
<extra environment> given in the constructor
(at the end of the scan, extra keys ‘endtime’ and ‘deadtime’ will be added representing the time at the end of the scan and the dead time)
This object is passed to all recorders at the beginning and at the end of the scan (when startRecordList and endRecordList is called)
At each step of the scan, for each Recorder, the writeRecord method will be called with a Record object as parameter. The Record.data member will be a dictionary containing:
‘point_nb’ : the point number of the scan
for each column of the scan (motor or counter), a key with the corresponding column name will contain the value
- takeSnapshot(elements=[])[source]
reads the current values of the given elements
- Parameters:
elements – (list<str,str>) list of tuples of label,src for the elements to read (can be pool elements or Taurus attribute names).
- Returns:
(list<ColumnDesc>) a list of
ColumnDesc
, each including a “pre_scan_value” attribute with the read value for that attr
- property data
Scan data.
- property generator
Generator of steps or waypoints used in this scan.
Scan
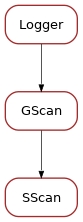
- class SScan(macro, generator=None, moveables=[], env={}, constraints=[], extrainfodesc=[])[source]
Bases:
GScan
Step scan
- property deterministic_scan
Check if the scan is a deterministic scan.
Scan is considered as deterministic scan if the
Macro
specialization owning the scan object containsnb_points
andinteg_time
attributes.Scan flow depends on this property (some optimizations are applied). These can be disabled by setting this property to
False
.
CScan
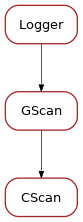
- class CScan(macro, generator=None, moveables=[], env={}, constraints=[], extrainfodesc=[])[source]
Bases:
GScan
Continuous scan abstract class. Implements helper methods.
- populate_moveables_data_structures(moveables)[source]
Populates moveables data structures. :type moveables: :param moveables: (list<Moveable>) data structures will be generated for these moveables :return (moveable_trees, physical_moveables_names, physical_moveables)
moveable_trees (list<Tree>) - each tree represent one Moveable with its hierarchy of inferior moveables.
physical_moveables_names (list<str> - list of the names of the physical moveables. List order is important and preserved.
physical_moveables (list<Moveable> - list of the moveable objects. List order is important and preserved.
- on_waypoints_end(restore_positions=None)[source]
To be called by the waypoint thread to handle the end of waypoints (either because no more waypoints or because a macro abort was triggered)
- get_velocity_range(motor)[source]
Helper method to find the max and min velocity for the motor. If the motor doesn’t have a defined range for velocity, it returns -inf and +inf
- get_max_top_velocity(motor)[source]
Helper method to find the maximum top velocity for the motor. If the motor doesn’t have a defined range for top velocity, then use the current top velocity
- get_max_velocity(motor)[source]
Helper method to find the maximum velocity for the motor. If the motor doesn’t have a defined range for max/min velocity, then return the current velocity
- get_min_velocity(motor)[source]
Helper method to find the minimum velocity for the motor. If the motor doesn’t have a defined range for max/min velocity, then use the current min velocity
- get_min_acc_time(motor)[source]
Helper method to find the minimum acceleration time for the motor. If the motor doesn’t have a defined range for the acceleration time, then use the current acceleration time
- get_min_dec_time(motor)[source]
Helper method to find the minimum deceleration time for the motor. If the motor doesn’t have a defined range for the acceleration time, then use the current acceleration time
- set_max_top_velocity(moveable)[source]
Helper method to set the maximum top velocity for the moveable to its maximum allowed limit.
- Parameters:
moveable (
BaseSardanaElement
) – moveable (motor or pseudo motor) on which to set the max top velocity- Return type:
- get_min_pos(motor)[source]
Helper method to find the minimum position for a given motor. If the motor doesn’t define its minimum position, then the negative infinite float representation is returned.