controller
This module contains the definition of the Controller base classes
Constants
- Type = 'type'
Constant data type (to be used as a key in the definition of
axis_attributes
orctrl_attributes
)
- Access = 'r/w type'
Constant data access (to be used as a key in the definition of
axis_attributes
orctrl_attributes
)
- Description = 'description'
Constant description (to be used as a key in the definition of
axis_attributes
orctrl_attributes
)
- DefaultValue = 'defaultvalue'
Constant default value (to be used as a key in the definition of
axis_attributes
orctrl_attributes
)
- FGet = 'fget'
Constant for getter function (to be used as a key in the definition of
axis_attributes
orctrl_attributes
)
- FSet = 'fset'
Constant for setter function (to be used as a key in the definition of
axis_attributes
orctrl_attributes
)
- Memorize = 'memorized'
Constant memorize (to be used as a key in the definition of
axis_attributes
orctrl_attributes
) Possible values for this key areMemorized
,MemorizedNoInit
andNotMemorized
- Memorized = 'true'
Constant memorized (to be used as a value in the
Memorize
field definition inaxis_attributes
orctrl_attributes
)
- MemorizedNoInit = 'true_without_hard_applied'
Constant memorize but not write at initialization (to be used as a value in the
Memorize
field definition inaxis_attributes
orctrl_attributes
)
- NotMemorized = 'false'
Constant not memorize (to be used as a value in the
Memorize
field definition inaxis_attributes
orctrl_attributes
)
- MaxDimSize = 'maxdimsize'
Constant MaxDimSize (to be used as a key in the definition of
axis_attributes
orctrl_attributes
)
Interfaces
Classes
Readable interface

- class Readable[source]
Bases:
object
A Readable interface. A controller for which it’s axis are ‘readable’ (like a motor, counter or 1D for example) should implement this interface
- PreReadAll()[source]
Controller API. Override if necessary. Called to prepare a read of the value of all axis. Default implementation does nothing.
- PreReadOne(axis)[source]
Controller API. Override if necessary. Called to prepare a read of the value of a single axis. Default implementation does nothing.
- Parameters:
axis (
int
) – axis number
- ReadAll()[source]
Controller API. Override if necessary. Called to read the value of all selected axis Default implementation does nothing.
- ReadOne(axis)[source]
Controller API. Override is MANDATORY! Default implementation raises
NotImplementedError
Referable interface

- class Referable[source]
Bases:
object
A Referable interface. A controller for which it’s axis can report data references (like a 1D or 2D for example) should implement this interface
Note
The Referable class has been included in Sardana on a provisional basis. Backwards incompatible changes (up to and including removal of the class) may occur if deemed necessary by the core developers.
- referable_axis_attributes = {'ValueRef': {'description': 'Value reference', 'r/w type': 0, 'type': <class 'str'>}, 'ValueRefBuffer': {'description': 'Value reference buffer', 'r/w type': 0, 'type': <class 'str'>}, 'ValueRefEnabled': {'description': 'Value reference enabled', 'r/w type': 1, 'type': <class 'bool'>}, 'ValueRefPattern': {'description': 'Value reference template', 'r/w type': 1, 'type': <class 'str'>}}
A
dict
containing the referable attributes present on each axis device
- RefOne(axis)[source]
Controller API. Override is MANDATORY! Default implementation raises
NotImplementedError
Startable interface

- class Startable[source]
Bases:
object
A Startable interface. A controller for which it’s axis are ‘startable’ (like a motor, for example) should implement this interface
- PreStartAll()[source]
Controller API. Override if necessary. Called to prepare a start of all axis (whatever pre-start means). Default implementation does nothing.
- PreStartOne(axis, value)[source]
Controller API. Override if necessary. Called to prepare a start of the given axis (whatever pre-start means). Default implementation returns True.
- StartOne(axis, value)[source]
Controller API. Override if necessary. Called to do a start of the given axis (whatever start means). Default implementation raises
NotImplementedError
Stopable interface

- class Stopable[source]
Bases:
object
A Stopable interface. A controller for which it’s axis are ‘stoppable’ (like a motor, for example) should implement this interface
- PreAbortAll()[source]
Controller API. Override if necessary. Called to prepare a abort of all axis (whatever pre-abort means). Default implementation does nothing.
- PreAbortOne(axis)[source]
Controller API. Override if necessary. Called to prepare a abort of the given axis (whatever pre-abort means). Default implementation returns True.
- AbortOne(axis)[source]
Controller API. Override is MANDATORY! Default implementation raises
NotImplementedError
. Aborts one of the axis- Parameters:
axis (
int
) – axis number
- AbortAll()[source]
Controller API. Override if necessary. Aborts all active axis of this controller. Default implementation does nothing.
- PreStopAll()[source]
Controller API. Override if necessary. Called to prepare a stop of all axis (whatever pre-stop means). Default implementation does nothing.
- PreStopOne(axis)[source]
Controller API. Override if necessary. Called to prepare a stop of the given axis (whatever pre-stop means). Default implementation returns True.
Loadable interface

- class Loadable[source]
Bases:
object
A Loadable interface. A controller for which it’s axis are ‘loadable’ (like a counter, 1D or 2D for example) should implement this interface
- default_timer = None
axis of the default timer
- PrepareOne(axis, value, repetitions, latency, nb_starts)[source]
Controller API. Override if necessary. Called to prepare the master channel axis with the measurement parameters. Default implementation does nothing.
- PreLoadAll()[source]
Controller API. Override if necessary. Called to prepare loading the integration time / monitor value. Default implementation does nothing.
- PreLoadOne(axis, value, repetitions, latency)[source]
Controller API. Override if necessary. Called to prepare loading the master channel axis with the acquisition parameters. Default implementation returns True.
- LoadAll()[source]
Controller API. Override if necessary. Called to load the integration time / monitor value. Default implementation does nothing.
- LoadOne(axis, value, repetitions, latency)[source]
Controller API. Override is MANDATORY! Called to load the integration time / monitor value. Default implementation raises
NotImplementedError
.
Synchronizer interface
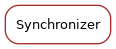
- class Synchronizer[source]
Bases:
object
A Synchronizer interface. A controller for which its axis are ‘Able to Synchronize’ should implement this interface
- PreSynchAll()[source]
Controller API. Override if necessary. Called to prepare loading the synchronization description. Default implementation does nothing.
- PreSynchOne(axis, description)[source]
Controller API. Override if necessary. Called to prepare loading the axis with the synchronization description. Default implementation returns True.
Abstract Controller

- class Controller(inst, props, *args, **kwargs)[source]
Bases:
object
Base controller class. Do NOT inherit from this class directly
- Parameters:
- ctrl_properties = {}
A
dict
containing controller properties where:key : (
str
) controller property namevalue :
dict
with with threestr
keys (“type”, “description” and “defaultvalue” case insensitive):for
Type
, value is one of the values described in Data Type definitionfor
Description
, value is astr
description of the property. if is not given it defaults to empty string.for
DefaultValue
, value is a python object or None if no default value exists for the property.
Example:
from sardana.pool.controller import MotorController, \ Type, Description, DefaultValue class MyCtrl(MotorController): ctrl_properties = \ { 'host' : { Type : str, Description : "host name" }, 'port' : { Type : int, Description : "port number", DefaultValue: 5000 } }
- ctrl_attributes = {}
A
dict
containning controller extra attributes where:key : (
str
) controller attribute namevalue :
dict
withstr
possible keys: “type”, “r/w type”, “description”, “fget”, “fset” and “maxdimsize” (case insensitive):for
Type
, value is one of the values described in Data Type definitionfor
Access
, value is one ofDataAccess
(“read” or “read_write” (case insensitive) strings are also accepted) [default: ReadWrite]for
Description
, value is astr
description of the attribute [default: “” (empty string)]for
FGet
, value is astr
with the method name for the attribute getter [default: “get”<controller attribute name>]for
FSet
, value is astr
with the method name for the attribute setter. [default, ifAccess
= “read_write”: “set”<controller attribute name>]for
DefaultValue
, value is a python object or None if no default value exists for the attribute. If given, the attribute is set when the controller is first created.for
Memorize
, value is astr
with possible values:Memorized
,MemorizedNoInit
andNotMemorized
[default:Memorized
]Added in version 1.1.
- for
MaxDimSize
, value is atuple
with possible values: for scalar must be an empty tuple ( () or [] ) [default: ()]
for 1D arrays a sequence with one value (example: (1024,)) [default: (2048,)]
for 1D arrays a sequence with two values (example: (1024, 1024)) [default: (2048, 2048)]
Added in version 1.1.
- for
Added in version 1.0.
Example:
from sardana.pool.controller import PseudoMotorController, \ Type, Description, DefaultValue, DataAccess class HKLCtrl(PseudoMotorController): ctrl_attributes = \ { 'ReflectionMatrix' : { Type : ( (float,), ), Description : "The reflection matrix", Access : DataAccess.ReadOnly, FGet : 'getReflectionMatrix', }, } def getReflectionMatrix(self): return ( (1.0, 0.0), (0.0, 1.0) )
- axis_attributes = {}
A
dict
containning controller extra attributes for each axis where:key : (
str
) axis attribute namevalue :
dict
with threestr
keys (“type”, “r/w type”, “description” case insensitive):for
Type
, value is one of the values described in Data Type definitionfor
Access
, value is one ofDataAccess
(“read” or “read_write” (case insensitive) strings are also accepted)for
Description
, value is astr
description of the attributefor
DefaultValue
, value is a python object or None if no default value exists for the attribute. If given, the attribute is set when the axis is first created.for
Memorize
, value is astr
with possible values:Memorized
,MemorizedNoInit
andNotMemorized
[default:Memorized
]Added in version 1.1.
- for
MaxDimSize
, value is atuple
with possible values: for scalar must be an empty tuple ( () or [] ) [default: ()]
for 1D arrays a sequence with one value (example: (1024,)) [default: (2048,)]
for 1D arrays a sequence with two values (example: (1024, 1024)) [default: (2048, 2048)]
Added in version 1.1.
- for
Added in version 1.0.
Example:
from sardana.pool.controller import MotorController, \ Type, Description, DefaultValue, DataAccess class MyMCtrl(MotorController): axis_attributes = \ { 'EncoderSource' : { Type : str, Description : 'motor encoder source', }, } def getAxisExtraPar(self, axis, name): name = name.lower() if name == 'encodersource': return self._encodersource[axis] def setAxisExtraPar(self, axis, name, value): name = name.lower() if name == 'encodersource': self._encodersource[axis] = value
- standard_axis_attributes = {}
A
dict
containing the standard attributes present on each axis device
- AddDevice(axis)[source]
Controller API. Override if necessary. Default implementation does nothing.
- Parameters:
axis (
int
) – axis number
- DeleteDevice(axis)[source]
Controller API. Override if necessary. Default implementation does nothing.
- Parameters:
axis (
int
) – axis number
- GetName()[source]
Controller API. The controller instance name.
- Return type:
- Returns:
the controller instance name
Added in version 1.0.
- GetAxisName(axis)[source]
Controller API. The axis name.
- Return type:
- Returns:
the axis name
Added in version 1.0.
- PreStateAll()[source]
Controller API. Override if necessary. Called to prepare a read of the state of all axis. Default implementation does nothing.
- PreStateOne(axis)[source]
Controller API. Override if necessary. Called to prepare a read of the state of a single axis. Default implementation does nothing.
- Parameters:
axis (
int
) – axis number
- StateAll()[source]
Controller API. Override if necessary. Called to read the state of all selected axis. Default implementation does nothing.
- StateOne(axis)[source]
Controller API. Override is MANDATORY. Called to read the state of one axis. Default implementation raises
NotImplementedError
.
- SetCtrlPar(parameter, value)[source]
Controller API. Override if necessary. Called to set a parameter with a value. Default implementation sets this object member named ‘_’+parameter with the given value.
Added in version 1.0.
- GetCtrlPar(parameter)[source]
Controller API. Override if necessary. Called to set a parameter with a value. Default implementation returns the value contained in this object’s member named ‘_’+parameter.
Added in version 1.0.
- SetAxisPar(axis, parameter, value)[source]
Controller API. Override is MANDATORY. Called to set a parameter with a value on the given axis. Default implementation raises
NotImplementedError
.Added in version 1.0.
- GetAxisPar(axis, parameter)[source]
Controller API. Override is MANDATORY. Called to get a parameter value on the given axis. Default implementation raises
NotImplementedError
.Added in version 1.0.
- SetAxisExtraPar(axis, parameter, value)[source]
Controller API. Override if necessary. Called to set a parameter with a value on the given axis. Default implementation raises
NotImplementedError
.Added in version 1.0.
- GetAxisExtraPar(axis, parameter)[source]
Controller API. Override if necessary. Called to get a parameter value on the given axis. Default implementation raises
NotImplementedError
.Added in version 1.0.
- GetAxisAttributes(axis)[source]
Controller API. Override if necessary. Returns a dictionary of all attributes per axis. Default implementation returns a new
dict
with the standard attributes plus theaxis_attributes
- Parameters:
axis (
int
) – axis number- Returns:
a dict containing attribute information as defined in
axis_attributes
Added in version 1.0.
- SendToCtrl(stream)[source]
Controller API. Override if necessary. Sends a string to the controller. Default implementation raises
NotImplementedError
.
Abstract Pseudo Controller
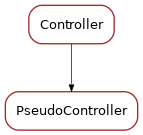
- class PseudoController(inst, props, *args, **kwargs)[source]
Bases:
Controller
Base class for all pseudo controllers.
Motor Controller API
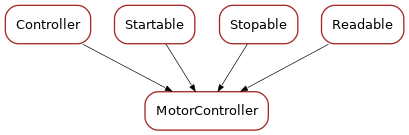
- class MotorController(inst, props, *args, **kwargs)[source]
Bases:
Controller
,Startable
,Stopable
,Readable
Base class for a motor controller. Inherit from this class to implement your own motor controller for the device pool.
A motor controller should support these axis parameters:
acceleration
deceleration
velocity
base_rate
step_per_unit
These parameters are configured through the
GetAxisPar()
/SetAxisPar()
API.- NoLimitSwitch = 0
A constant representing no active switch.
- HomeLimitSwitch = 1
A constant representing an active home switch. You can OR two or more switches together. For example, to say both upper and lower limit switches are active:
limit_switches = self.HomeLimitSwitch | self.LowerLimitSwitch
- UpperLimitSwitch = 2
A constant representing an active upper limit switch. You can OR two or more switches together. For example, to say both upper and lower limit switches are active:
limit_switches = self.UpperLimitSwitch | self.LowerLimitSwitch
- LowerLimitSwitch = 4
A constant representing an active lower limit switch. You can OR two or more switches together. For example, to say both upper and lower limit switches are active:
limit_switches = self.UpperLimitSwitch | self.LowerLimitSwitch
- standard_axis_attributes = {'Acceleration': {'description': 'Acceleration time (s)', 'type': <class 'float'>}, 'Backlash': {'description': 'Backlash', 'type': <class 'float'>}, 'Base_rate': {'description': 'Base rate', 'type': <class 'float'>}, 'Deceleration': {'description': 'Deceleration time (s)', 'type': <class 'float'>}, 'DialPosition': {'description': 'Dial Position', 'type': <class 'float'>}, 'Limit_switches': {'description': "This attribute is the motor limit switches state. It's an array with 3 \nelements which are:\n0 - The home switch\n1 - The upper limit switch\n2 - The lower limit switch\nFalse means not active. True means active", 'type': (<class 'bool'>,)}, 'Offset': {'description': 'Offset', 'type': <class 'float'>}, 'Position': {'description': 'Position', 'type': <class 'float'>}, 'Sign': {'description': 'Sign', 'type': <class 'float'>}, 'Step_per_unit': {'description': 'Steps per unit', 'type': <class 'float'>}, 'Velocity': {'description': 'Velocity', 'type': <class 'float'>}}
A
dict
containing the standard attributes present on each axis device
- GetAxisAttributes(axis)[source]
Motor Controller API. Override if necessary. Returns a sequence of all attributes per axis. Default implementation returns a
dict
containning:Position
DialPosition
Offset
Sign
Step_per_unit
Acceleration
Deceleration
Base_rate
Velocity
Backlash
Limit_switches
plus all attributes contained in
axis_attributes
Note
Normally you don’t need to Override this method. You just implement the class member
axis_attributes
. Typically, you will need to Override this method in two cases:certain axes contain a different set of extra attributes which cannot be simply defined in
axis_attributes
some axes (or all) don’t implement a set of standard moveable parameters (ex.: if a motor controller is created to control a power supply, it may have a position (current) and a velocity (ramp speed) but it may not have acceleration)
- Parameters:
axis (
int
) – axis number- Returns:
a dict containing attribute information as defined in
axis_attributes
Added in version 1.0.
Pseudo Motor Controller API
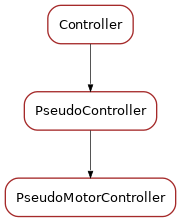
- class PseudoMotorController(inst, props, *args, **kwargs)[source]
Bases:
PseudoController
Base class for a pseudo motor controller. Inherit from this class to implement your own pseudo motor controller for the device pool.
Every Pseudo Motor implementation must be a subclass of this class. Current procedure for a correct implementation of a Pseudo Motor class:
- mandatory:
define the class level attributes
pseudo_motor_roles
,motor_roles
write
CalcPseudo()
methodwrite
CalcPhysical()
method.
- optional:
write
CalcAllPseudo()
andCalcAllPhysical()
if great performance gain can be achived
- pseudo_motor_roles = ()
a sequence of strings describing the role of each pseudo motor axis in this controller
- motor_roles = ()
a sequence of strings describing the role of each motor in this controller
- standard_axis_attributes = {'Position': {'description': 'Position', 'type': <class 'float'>}}
A
dict
containing the standard attributes present on each axis device
- CalcAllPseudo(physical_pos, curr_pseudo_pos)[source]
Pseudo Motor Controller API. Override if necessary. Calculates the positions of all pseudo motors that belong to the pseudo motor system from the positions of the physical motors. Default implementation does a loop calling
PseudoMotorController.calc_pseudo()
for each pseudo motor role.- Parameters:
- Return type:
- Returns:
a sequece of pseudo motor positions (one for each pseudo motor role)
Added in version 1.0.
- CalcAllPhysical(pseudo_pos, curr_physical_pos)[source]
Pseudo Motor Controller API. Override if necessary. Calculates the positions of all motors that belong to the pseudo motor system from the positions of the pseudo motors. Default implementation does a loop calling
PseudoMotorController.calc_physical()
for each motor role.- Parameters:
- Return type:
- Returns:
a sequece of motor positions (one for each motor role)
Added in version 1.0.
- CalcPseudo(axis, physical_pos, curr_pseudo_pos)[source]
Pseudo Motor Controller API. Override is MANDATORY. Calculate pseudo motor position given the physical motor positions
- Parameters:
- Return type:
- Returns:
a pseudo motor position corresponding to the given axis pseudo motor role
Added in version 1.0.
- CalcPhysical(axis, pseudo_pos, curr_physical_pos)[source]
Pseudo Motor Controller API. Override is MANDATORY. Calculate physical motor position given the pseudo motor positions.
- Parameters:
- Return type:
- Returns:
a motor position corresponding to the given axis motor role
Added in version 1.0.
- GetMotor(index_or_role)[source]
Returns the motor for a given role/index.
Warning
Use with care: Executing motor methods can be dangerous!
Since the controller is built before any element (including motors), this method will FAIL when called from the controller constructor
- GetPseudoMotor(index_or_role)[source]
Returns the pseudo motor for a given role/index.
Warning
Use with care: Executing pseudo motor methods can be dangerous!
Since the controller is built before any element (including pseudo motors), this method will FAIL when called from the controller constructor
- Parameters:
- Return type:
- Returns:
PseudoMotor object for the given role/index
Counter Timer Controller API
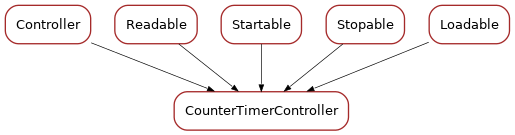
- class CounterTimerController(inst, props, *args, **kwargs)[source]
Bases:
Controller
,Readable
,Startable
,Stopable
,Loadable
Base class for a counter/timer controller. Inherit from this class to implement your own counter/timer controller for the device pool.
A counter timer controller should support these controller parameters:
timer
monitor
- standard_axis_attributes = {'IntegrationTime': {'description': 'Integration time used in independent acquisition', 'type': <class 'float'>}, 'Shape': {'description': 'Shape of the value, it is an empty array', 'type': (<class 'int'>,)}, 'Timer': {'description': 'Timer used in independent acquisition', 'type': <class 'str'>}, 'Value': {'description': 'Value', 'type': <class 'float'>}, 'ValueBuffer': {'description': 'Value buffer', 'type': <class 'str'>}}
A
dict
containing the standard attributes present on each axis device
0D Controller API
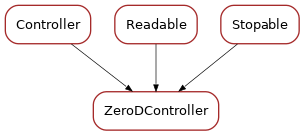
- class ZeroDController(inst, props, *args, **kwargs)[source]
Bases:
Controller
,Readable
,Stopable
Base class for a 0D controller. Inherit from this class to implement your own 0D controller for the device pool.
- standard_axis_attributes = {'IntegrationTime': {'description': 'Integration time used in independent acquisition', 'type': <class 'float'>}, 'Shape': {'description': 'Shape of the value, it is an empty array', 'type': (<class 'int'>,)}, 'Value': {'description': 'Value', 'type': <class 'float'>}, 'ValueBuffer': {'description': 'Value buffer', 'type': <class 'str'>}}
A
dict
containing the standard attributes present on each axis device
1D Controller API
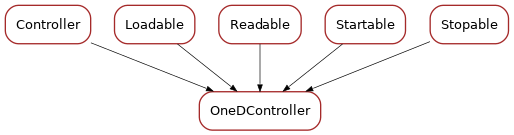
- class OneDController(inst, props, *args, **kwargs)[source]
Bases:
Controller
,Readable
,Startable
,Stopable
,Loadable
Base class for a 1D controller. Inherit from this class to implement your own 1D controller for the device pool.
Added in version 1.2.
- standard_axis_attributes = {'IntegrationTime': {'description': 'Integration time used in independent acquisition', 'type': <class 'float'>}, 'Shape': {'description': 'Shape of the value, it is an array with 1 element - X dimension', 'type': (<class 'int'>,)}, 'Timer': {'description': 'Timer used in independent acquisition', 'type': <class 'str'>}, 'Value': {'description': 'Value', 'maxdimsize': (16384,), 'type': (<class 'float'>,)}, 'ValueBuffer': {'description': 'Value buffer', 'type': <class 'str'>}}
A
dict
containing the standard attributes present on each axis device
- GetAxisPar(axis, parameter)[source]
Controller API. Override is MANDATORY. Called to get a parameter value on the given axis.
GetAxisPar
with ‘data_source’ parameter is deprecated since 2.8.0. Inherit fromReferable
class in order to report value references.Default implementation calls deprecated
GetPar()
which, by default, raisesNotImplementedError
.Added in version 1.2.
2D Controller API
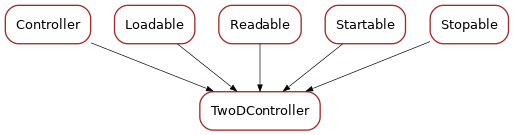
- class TwoDController(inst, props, *args, **kwargs)[source]
Bases:
Controller
,Readable
,Startable
,Stopable
,Loadable
Base class for a 2D controller. Inherit from this class to implement your own 2D controller for the device pool.
- standard_axis_attributes = {'IntegrationTime': {'description': 'Integration time used in independent acquisition', 'type': <class 'float'>}, 'Shape': {'description': 'Shape of the value, it is an array with 2 elements: X and Y dimensions', 'type': (<class 'int'>,)}, 'Timer': {'description': 'Timer used in independent acquisition', 'type': <class 'str'>}, 'Value': {'description': 'Value', 'maxdimsize': (4096, 4096), 'type': ((<class 'float'>,),)}, 'ValueBuffer': {'description': 'Value buffer', 'type': <class 'str'>}}
A
dict
containing the standard attributes present on each axis device
- GetAxisPar(axis, parameter)[source]
Controller API. Override is MANDATORY. Called to get a parameter value on the given axis.
GetAxisPar
with ‘data_source’ parameter is deprecated since 2.8.0. Inherit fromReferable
class in order to report value references.Default implementation calls deprecated
GetPar()
which, by default, raisesNotImplementedError
.Added in version 1.2.
Pseudo Counter Controller API
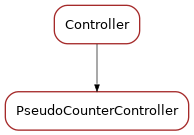
- class PseudoCounterController(inst, props, *args, **kwargs)[source]
Bases:
Controller
Base class for a pseudo counter controller. Inherit from this class to implement your own pseudo counter controller for the device pool.
Every Pseudo Counter implementation must be a subclass of this class. Current procedure for a correct implementation of a Pseudo Counter class:
- mandatory:
define the class level attributes
counter_roles
,write
Calc()
method
- pseudo_counter_roles = ()
a sequence of strings describing the role of each pseudo counter axis in this controller
- counter_roles = ()
a sequence of strings describing the role of each counter in this controller
- standard_axis_attributes = {'IntegrationTime': {'description': 'Integration time used in independent acquisition', 'type': <class 'float'>}, 'Shape': {'description': 'Shape of the value, it is an empty array', 'type': (<class 'int'>,)}, 'Value': {'description': 'Value', 'type': <class 'float'>}, 'ValueBuffer': {'description': 'Data', 'type': <class 'str'>}}
A
dict
containing the standard attributes present on each axis device
- Calc(axis, values)[source]
Pseudo Counter Controller API. Override is MANDATORY. Calculate pseudo counter position given the counter values.
- Parameters:
- Return type:
- Returns:
a pseudo counter value corresponding to the given axis pseudo counter role
Added in version 1.0.
- CalcAll(values)[source]
Pseudo Counter Controller API. Override if necessary. Calculates all pseudo counter values from the values of counters. Default implementation does a loop calling
PseudoCounterController.Calc()
for each pseudo counter role.- Parameters:
values (
Sequence
[float
]) – a sequence containing current values of underlying elements- Return type:
- Returns:
a sequece of pseudo counter values (one for each pseudo counter role)
Added in version 1.2.
Trigger/Gate Controller API
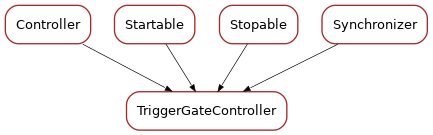
- class TriggerGateController(inst, props, *args, **kwargs)[source]
Bases:
Controller
,Synchronizer
,Stopable
,Startable
Base class for a trigger/gate controller. Inherit from this class to implement your own trigger/gate controller for the device pool.
IO Register Controller API
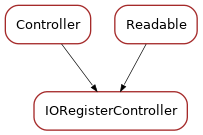
- class IORegisterController(inst, props, *args, **kwargs)[source]
Bases:
Controller
,Readable
Base class for a IORegister controller. Inherit from this class to implement your own IORegister controller for the device pool.