PoolDevice
Generic Tango Pool Device base classes
Classes
PoolDevice
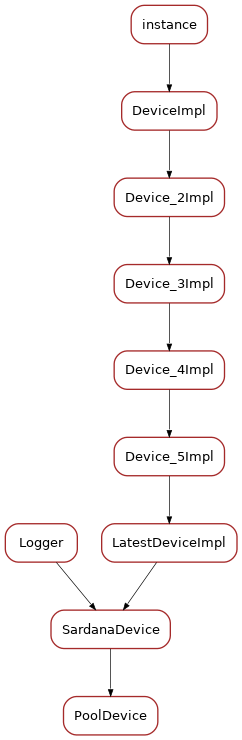
- class PoolDevice(dclass, name)[source]
Bases:
SardanaDevice
Base Tango Pool device class
- ExtremeErrorStates = (tango._tango.DevState.FAULT, tango._tango.DevState.UNKNOWN)
list of extreme error states
- BusyStates = (tango._tango.DevState.MOVING, tango._tango.DevState.RUNNING)
list of busy states
- BusyRetries = 3
Maximum number of retries in a busy state
- init(name)[source]
initialize the device once in the object lifetime. Override when necessary but always call the method from your super class
- Parameters:
name (
str
) – device name
- property pool_device
The tango pool device
- property pool
The sardana pool object
- get_element()[source]
Returns the underlying pool element object
- Return type:
- Returns:
the underlying pool element object
- set_element(element)[source]
Associates this device with the sardana element
- Parameters:
element (
PoolElement
) – the sardana element- Return type:
- property element: PoolElement
The underlying sardana element
- init_device()[source]
Initialize the device. Called during startup after
init()
and every time the tangoInit
command is executed. Override when necessary but always call the method from your super class
- delete_device()[source]
Clean the device. Called during shutdown and every time the tango
Init
command is executed. Override when necessary but always call the method from your super class
- is_Abort_allowed()[source]
Returns True if it is allowed to execute the tango abort command
- Return type:
- Returns:
True if it is allowed to execute the tango abort command or False otherwise
- is_Stop_allowed()[source]
Returns True if it is allowed to execute the tango stop command
- Return type:
- Returns:
True if it is allowed to execute the tango stop command or False otherwise
- is_Release_allowed()[source]
Returns True if it is allowed to execute the tango release command
- Return type:
- Returns:
True if it is allowed to execute the tango release command or False otherwise
- get_dynamic_attributes()[source]
Returns the standard dynamic and fully dynamic attributes for this device. The return is a tuple of two dictionaries:
standard attributes: caseless dictionary with key being the attribute name and value is a tuple of attribute name(str), tango information, attribute information
dynamic attributes: caseless dictionary with key being the attribute name and value is a tuple of attribute name(str), tango information, attribute information
- tango information
seq<
CmdArgType
,AttrDataFormat
,AttrWriteType
>- attribute information
attribute information as returned by the sardana controller
- Return type:
- Returns:
the standard dynamic and fully dynamic attributes
- remove_unwanted_dynamic_attributes(new_std_attrs, new_dyn_attrs)[source]
Removes unwanted dynamic attributes from previous device creation
- add_dynamic_attribute(attr_name, data_info, attr_info, read, write, is_allowed)[source]
Adds a single dynamic attribute
- Parameters:
- Return type:
Attr
- add_standard_attribute(attr_name, data_info, attr_info, read, write, is_allowed)[source]
Adds a single standard dynamic attribute
- Parameters:
- Return type:
Attr
- read_DynamicAttribute(attr)[source]
Generic read dynamic attribute. Default implementation raises
NotImplementedError
- Parameters:
attr (
Attribute
) – attribute to be read- Raises:
- Return type:
- write_DynamicAttribute(attr)[source]
Generic write dynamic attribute. Default implementation raises
NotImplementedError
- Parameters:
attr (
Attribute
) – attribute to be written- Raises:
- Return type:
- is_DynamicAttribute_allowed(req_type)[source]
Generic is dynamic attribute allowed. Default implementation calls
_is_allowed()
- dev_state()[source]
Calculates and returns the device state. Called by Tango on a read state request.
- Return type:
DevState
- Returns:
the device state
- dev_status()[source]
Calculates and returns the device status. Called by Tango on a read status request.
- Return type:
- Returns:
the device status
- wait_for_operation()[source]
Waits for an operation to finish. It uses the maxumum number of retries. Sleeps 0.01s between retries.
- Raises:
Exception
in case of a timeout
PoolDeviceClass
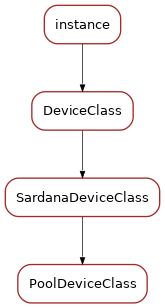
- class PoolDeviceClass(name)[source]
Bases:
SardanaDeviceClass
Base Tango Pool Device Class class
- class_property_list = {}
Sardana device class properties definition
- device_property_list = {'Force_HW_Read': [tango._tango.CmdArgType.DevBoolean, 'Force a hardware read of value even when in operation (motion/acquisition', False], 'Id': [tango._tango.CmdArgType.DevLong64, 'Internal ID', 0]}
Sardana device properties definition
- cmd_list = {'Abort': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']], 'Release': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']], 'Restore': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']], 'Stop': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']]}
Sardana device command definition
- attr_list = {}
Sardana device attribute definition
- standard_attr_list = {}
PoolElementDevice
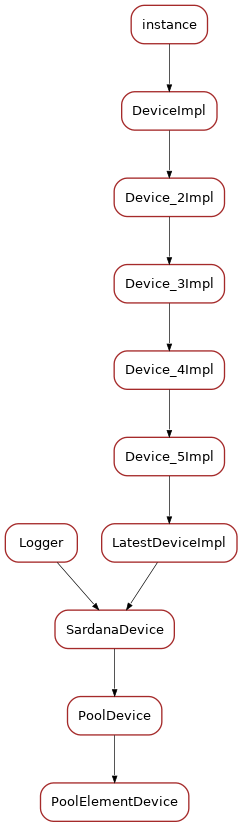
- class PoolElementDevice(dclass, name)[source]
Bases:
PoolDevice
Base Tango Pool Element Device class
- ignore_memorized_attrs_during_initialization = ()
- init_device()[source]
Initialize the device. Called during startup after
init()
and every time the tangoInit
command is executed. Override when necessary but always call the method from your super class
- delete_device()[source]
Clean the device. Called during shutdown and every time the tango
Init
command is executed. Override when necessary but always call the method from your super class
- read_Instrument(attr)[source]
Read the value of the
Instrument
tango attribute. Returns the instrument full name or empty string if this element doesn’t belong to any instrument- Parameters:
attr (
Attribute
) – tango instrument attribute- Return type:
- write_Instrument(attr)[source]
Write the value of the
Instrument
tango attribute. Sets a new instrument full name or empty string if this element doesn’t belong to any instrument. The instrument must have been previously created.- Parameters:
attr (
Attribute
) – tango instrument attribute- Return type:
- get_dynamic_attributes()[source]
Override of
PoolDevice.get_dynamic_attributes
. Returns the standard dynamic and fully dynamic attributes for this device. The return is a tuple of two dictionaries:standard attributes: caseless dictionary with key being the attribute name and value is a tuple of attribute name(str), tango information, attribute information
dynamic attributes: caseless dictionary with key being the attribute name and value is a tuple of attribute name(str), tango information, attribute information
- tango information
seq<
CmdArgType
,AttrDataFormat
,AttrWriteType
>- attribute information
attribute information as returned by the sardana controller
- Return type:
- Returns:
the standard dynamic and fully dynamic attributes
- read_DynamicAttribute(attr)[source]
Read a generic dynamic attribute. Calls the controller of this element to get the dynamic attribute value
- Parameters:
attr (
Attribute
) – tango attribute- Return type:
- write_DynamicAttribute(attr)[source]
Write a generic dynamic attribute. Calls the controller of this element to get the dynamic attribute value
- Parameters:
attr (
Attribute
) – tango attribute- Return type:
PoolElementDeviceClass
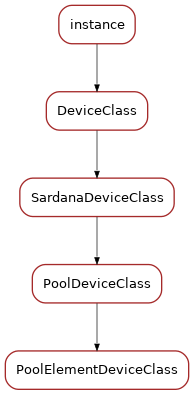
- class PoolElementDeviceClass(name)[source]
Bases:
PoolDeviceClass
Base Tango Pool Element Device Class class
- device_property_list = {'Axis': [tango._tango.CmdArgType.DevLong64, 'Axis in the controller', [0]], 'Ctrl_id': [tango._tango.CmdArgType.DevString, 'Controller ID', [0]], 'Force_HW_Read': [tango._tango.CmdArgType.DevBoolean, 'Force a hardware read of value even when in operation (motion/acquisition', False], 'Id': [tango._tango.CmdArgType.DevLong64, 'Internal ID', 0], 'Instrument_id': [tango._tango.CmdArgType.DevString, 'Instrument ID', [0]]}
Sardana device properties definition
- attr_list = {'Instrument': [[tango._tango.CmdArgType.DevString, tango._tango.AttrDataFormat.SCALAR, tango._tango.AttrWriteType.READ_WRITE], {'Display level': tango._tango.DispLevel.EXPERT, 'label': 'Instrument'}], 'SimulationMode': [[tango._tango.CmdArgType.DevBoolean, tango._tango.AttrDataFormat.SCALAR, tango._tango.AttrWriteType.READ_WRITE], {'label': 'Simulation mode'}]}
Sardana device attribute definition
- cmd_list = {'Abort': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']], 'Release': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']], 'Restore': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']], 'Stop': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']]}
Sardana device command definition
PoolGroupDevice
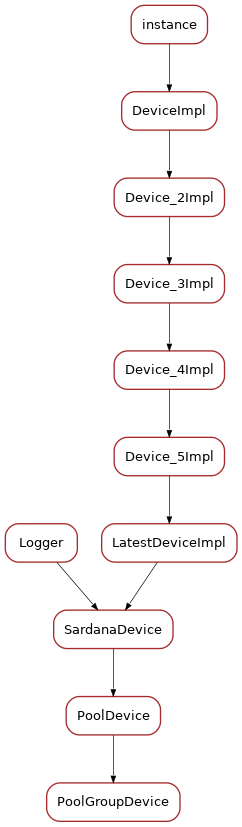
- class PoolGroupDevice(dclass, name)[source]
Bases:
PoolDevice
Base Tango Pool Group Device class
PoolGroupDeviceClass
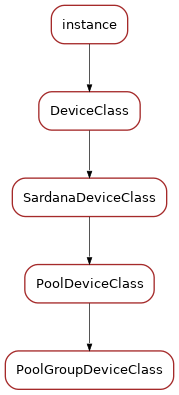
- class PoolGroupDeviceClass(name)[source]
Bases:
PoolDeviceClass
Base Tango Pool Group Device Class class
- device_property_list = {'Elements': [tango._tango.CmdArgType.DevVarStringArray, 'elements in the group', []], 'Force_HW_Read': [tango._tango.CmdArgType.DevBoolean, 'Force a hardware read of value even when in operation (motion/acquisition', False], 'Id': [tango._tango.CmdArgType.DevLong64, 'Internal ID', 0]}
Sardana device properties definition
- cmd_list = {'Abort': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']], 'Release': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']], 'Restore': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']], 'Stop': [[tango._tango.CmdArgType.DevVoid, ''], [tango._tango.CmdArgType.DevVoid, '']]}
Sardana device command definition
- attr_list = {'ElementList': [[tango._tango.CmdArgType.DevString, tango._tango.AttrDataFormat.SPECTRUM, tango._tango.AttrWriteType.READ, 4096]]}
Sardana device attribute definition